A rather tedious, but quite common, task in nearly every application is to prompt the user for filenames, font names and sizes, or colors to be used by the application. Designing your own dialog boxes for these purposes would be a hassle, not to mention that your applications wouldn’t conform to the basic Windows interface design principles. In fact, all Windows applications use standard dialog boxes for common operations; two of them are shown in Figure 4.10. These dialog boxes are implemented as standard controls in the Toolbox. To use any of the common dialog controls in your interface, just place the appropriate control from the Dialog section of the Toolbox on your form and activate it from within your code by calling the ShowDialog method.
The common dialog controls are invisible at runtime, and they’re not placed on your forms, because they’re implemented as modal dialog boxes and they’re displayed as needed. You simply add them to the project by double-clicking their icons in the Toolbox; a new icon appears in the components tray of the form, just below the Form Designer. The common dialog controls in the Toolbox are the following:
- OpenFileDialog – Lets users select a file to open. It also allows the selection of multiple files for applications that must process many files at once.
- SaveFileDialog – Lets users select or specify the path of a file in which the current document will be saved.
- FolderBrowserDialog – Lets users select a folder (an operation that can’t be performed with the OpenFileDialog control).
- ColorDialog – Lets users select a color from a list of predefined colors or specify custom colors. FontDialog Lets users select a typeface and style to be applied to the current text selection. The Font dialog box has an Apply button, which you can intercept from within your code and use to apply the currently selected font to the text without closing the dialog box.
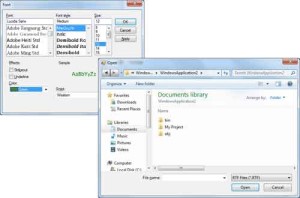
Figure 4.10 – The Open and Font common dialog boxes
There are three more common dialog controls: the PrintDialog, PrintPreviewDialog, and PageSetupDialog controls. These controls are discussed in detail in Chapter, “Printing with Visual Basic 2008,” in the context of VB’s printing capabilities.
Using the Common Dialog Controls
To display any of the common dialog boxes from within your application, you must first add an instance of the appropriate control to your project. Then you must set some basic properties of the control through the Properties window. Most applications set the control’s properties from within the code because common dialogs interact closely with the application. When you call the Color common dialog, for example, you should preselect a color from within your application and make it the default selection on the control. When prompting the user for the color of the text, the default selection should be the current setting of the control’s ForeColor property. Likewise, the Save dialog box must suggest a filename when it first pops up (or the file’s extension, at least).
To display a common dialog box from within your code, you simply call the control’s ShowDialog method, which is common for all controls. Note that all common dialog controls can be displayed only modally and they don’t expose a Show method. As soon as you call the ShowDialog method, the corresponding dialog box appears onscreen, and the execution of the program is suspended until the box is closed. Using the Open, Save, and FolderBrowser dialog boxes, users can traverse the entire structure of their drives and locate the desired filename or folder. When the user clicks the Open or Save button, the dialog box closes and the program’s execution resumes. The code should read the name of the file selected by the user through the FileName property and use it to open the file or store the current document there. The folder selected in the FolderBrowserDialog control is returned to the application through the SelectedPath property.
Here is the sequence of statements used to invoke the Open common dialog and retrieve the selected filename:
If OpenFileDialog1.ShowDialog = Windows.Forms.DialogResult.OK Then
fileName = OpenFileDialog1.FileName
' Statements to open the selected file
End If
Code language: VB.NET (vbnet)
The ShowDialog method returns a value indicating how the dialog box was closed. You should read this value from within your code and ignore the settings of the dialog box if the operation was cancelled.
The variable fileName in the preceding code segment is the full pathname of the file selected by the user. You can also set the FileName property to a filename, which will be displayed when the Open dialog box is first opened:
OpenFileDialog1.FileName = "C:\WorkFiles\Documents\Document1.doc"
If OpenFileDialog1.ShowDialog = Windows.Forms.DialogResult.OK Then
fileName = OpenFileDialog1.FileName
' Statements to open the selected file
End If
Code language: VB.NET (vbnet)
Similarly, you can invoke the Color dialog box and read the value of the selected color by using the following statements:
ColorDialog1.Color = TextBox1.BackColor
If ColorDialog1.ShowDialog = DialogResult.OK Then
TextBox1.BackColor = ColorDialog1.Color
End If
Code language: VB.NET (vbnet)
The ShowDialog method is common to all controls. The Title property is also common to all controls and it’s the string displayed in the title bar of the dialog box. The default title is the name of the dialog box (for example, Open, Color, and so on), but you can adjust it from within your code with a statement such as the following:
ColorDialog1.Title = "Select Drawing Color"
Code language: VB.NET (vbnet)