The Font dialog box, shown in Figure 4.12, lets the user review and select a font and then set its size and style. Optionally, users can also select the font’s color and even apply the current settings to the selected text on a control of the form without closing the dialog box, by clicking the Apply button.
When the dialog is closed by clicking the OK button, you can retrieve the selected font by using the control’s Font property. In addition to the OK button, the Font dialog box may contain the Apply button, which reports the current setting to your application. You can intercept the Click event of the Apply button and adjust the appearance of the text on your form while the common dialog is still visible.
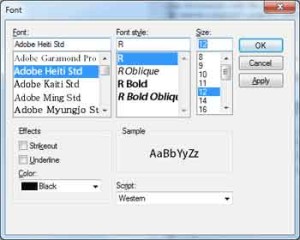
Figure 4.12 – Font dialog box in VB2008
The main property of this control is the Font property, which sets the initially selected font in the dialog box and retrieves the font selected by the user. The following statements display the Font dialog box after setting the initial font to the current font of the TextBox1 control. When the user closes the dialog box, the code retrieves the selected font and assigns it to the same TextBox control:
FontDialog1.Font = TextBox1.Font
If FontDialog1.ShowDialog = DialogResult.OK Then
TextBox1.Font = FontDialog1.Font
End If
Code language: VB.NET (vbnet)
Use the following properties to customize the Font dialog box before displaying it.
AllowScriptChange
This property is a Boolean value that indicates whether the Script combo box will be displayed in the Font dialog box. This combo box allows the user to change the current character set and select a non-Western language (such as Greek, Hebrew, Cyrillic, and so on).
AllowVerticalFonts
This property is a Boolean value that indicates whether the dialog box allows the display and selection of both vertical and horizontal fonts. Its default value is False, which displays only horizontal fonts.
Color, ShowColor
The Color property sets or returns the selected font color. To enable users to select a color for the font, you must also set the ShowColor property to True.
FixedPitchOnly
This property is a Boolean value that indicates whether the dialog box allows only the selection of fixed-pitch fonts. Its default value is False, which means that all fonts (fixed- and variable-pitch fonts) are displayed in the Font dialog box. Fixed-pitch fonts, or monospaced fonts, consist of characters of equal widths that are sometimes used to display columns of numeric values so that the digits are aligned vertically.
Font
This property is a Font object. You can set it to the preselected font before displaying the dialog box and assign it to a Font property upon return. You’ve already seen how to preselect a font and how to apply the selected font to a control from within your application.
You can also create a new Font object and assign it to the control’s Font property. Upon return, the TextBox control’s Font property is set to the selected font:
Dim newFont As Font("Verdana", 12, FontStyle.Underline)
FontDialog1.Font = newFont
If FontDialog1.ShowDialog() = DialogResult.OK Then
TextBox1.ForeColor = FontDialog1.Color
End If
Code language: VB.NET (vbnet)
FontMustExist
This property is a Boolean value that indicates whether the dialog box forces the selection of an existing font. If the user enters a font name that doesn’t correspond to a name in the list of available fonts, a warning is displayed. Its default value is True, and there’s no reason to change it.
MaxSize, MinSize
These two properties are integers that determine the minimum and maximum point size the user can specify in the Font dialog box. Use these two properties to prevent the selection of extremely large or extremely small font sizes, because these fonts might throw off a well-balanced interface (text will overflow in labels, for example).
ShowApply
This property is a Boolean value that indicates whether the dialog box provides an Apply button. Its default value is False, so the Apply button isn’t normally displayed. If you set this property to True, you must also program the control’s Apply event — the changes aren’t applied automatically to any of the controls in the current form.
The following statements display the Font dialog box with the Apply button:
Private Sub Button2 Click(...) Handles Button2.Click
FontDialog1.Font = TextBox1.Font
FontDialog1.ShowApply = True
If FontDialog1.ShowDialog = DialogResult.OK Then
TextBox1.Font = FontDialog1.Font
End If
End Sub
Code language: VB.NET (vbnet)
The FontDialog control raises the Apply event every time the user clicks the Apply button. In this event’s handler, you must read the currently selected font and use it in the form, so that users can preview the effect of their selection:
Private Sub FontDialog1 Apply(...) Handles FontDialog1.Apply
TextBox1.Font = FontDialog1.Font
End Sub
Code language: VB.NET (vbnet)
ShowEffects
This property is a Boolean value that indicates whether the dialog box allows the selection of special text effects, such as strikethrough and underline. The effects are returned to the application as attributes of the selected Font object, and you don’t have to do anything special in your application.