The Color dialog box, shown in Figure 4.11, is one of the simplest dialog boxes. Its Color property returns the color selected by the user or sets the initially selected color when the user opens the dialog box.
The following statements set the initial color of the ColorDialog control, display the dialog box, and then use the color selected in the control to fill the form. First, place a ColorDialog control in the form and then insert the following statements in a button’s Click event handler:
Private Sub Button1 Click(...) Handles Button1.Click
ColorDialog1.Color = Me.BackColor
If ColorDialog1.ShowDialog = Windows.Forms.DialogResult.OK Then
Me.BackColor = ColorDialog1.Color
End If
End Sub
Code language: VB.NET (vbnet)
The following sections discuss the basic properties of the ColorDialog control.
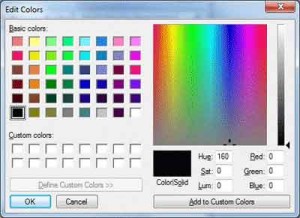
Figure 4.11 – The Color Dialog Box
AllowFullOpen
Set this property to True if you want users to be able to open the dialog box and define their own custom colors, like the one shown in Figure 8.2. The AllowFullOpen property doesn’t open the custom section of the dialog box; it simply enables the Define Custom Colors button in the dialog box. Otherwise, this button is disabled.
AnyColor
This property is a Boolean value that determines whether the dialog box displays all available colors in the set of basic colors.
Color
This is the color specified on the control. You can set it to a color value before showing the dialog box to suggest a reasonable selection. On return, read the value of the same property to find out which color was picked by the user in the control:
ColorDialog1.Color = Me.BackColor
If ColorDialog1.ShowDialog = DialogResult.OK Then
Me.BackColor = ColorDialog1.Color
End If
Code language: VB.NET (vbnet)
CustomColors
This property indicates the set of custom colors that will be shown in the dialog box. The Color dialog box has a section called Custom Colors, in which you can display 16 additional custom colors. The CustomColors property is an array of integers that represent colors. To display three custom colors in the lower section of the Color dialog box, use a statement such as the following:
Dim colors() As Integer = {222663, 35453, 7888}
ColorDialog1.CustomColors = colors
Code language: VB.NET (vbnet)
You’d expect that the CustomColors property would be an array of Color values, but it’s not. You can’t create the array CustomColors with a statement such as this one:
Dim colors() As Color = _
{Color.Azure, Color.Navy, Color.Teal}
Code language: VB.NET (vbnet)
Because it’s awkward to work with numeric values, you should convert color values to integer values by using a statement such as the following:
Color.Navy.ToArgb
The preceding statement returns an integer value that represents the color navy. This value, however, is negative because the first byte in the color value represents the transparency of the color. To get the value of the color, you must take the absolute value of the integer value returned by the previous expression. To create an array of integers that represent color values, use a statement such as the following:
Dim colors() As Integer = _
{Math.Abs(Color.Gray.ToArgb), _
Math.Abs(Color.Navy.ToArgb), _
Math.Abs(Color.Teal.ToArgb)}
Code language: VB.NET (vbnet)
Now you can assign the colors array to the CustomColors property of the control, and the colors will appear in the Custom Colors section of the Color dialog box.
SolidColorOnly
This indicates whether the dialog box will restrict users to selecting solid colors only. This setting should be used with systems that can display only 256 colors. Although today few systems can’t display more than 256 colors, some interfaces are limited to this number. When you run an application through Remote Desktop, for example, only the solid colors are displayed correctly on the remote screen, regardless of the remote computer’s graphics card (and that’s for efficiency reasons).