Open and Save As, the two most widely used common dialog boxes (see Figure 4.13), are implemented by the OpenFileDialog and SaveFileDialog controls. Nearly every application prompts users for filenames, and the .NET Framework provides two controls for this purpose. The two dialog boxes are nearly identical, and most of their properties are common, so we’ll start with the properties that are common to both controls.
When either of the two controls is displayed, it rarely displays all the files in any given folder. Usually the files displayed are limited to the ones that the application recognizes so that users can easily spot the file they want. The Filter property limits the types of files that will appear in the Open or Save As dialog box.
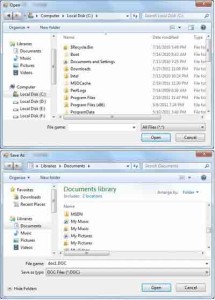
Figure 4.13 – The Open and Save As common dialog boxes
It’s also standard for the Windows interface not to display the extensions of files (although Windows distinguishes files by their extensions). The file type ComboBox, which appears at the bottom of the form next to the File Name box, contains the various file types recognized by the application. The various file types can be described in plain English with long descriptive names and without their extensions.
The extension of the default file type for the application is described by the DefaultExtension property, and the list of the file types displayed in the Save As Type box is determined by the Filter property.
To prompt the user for a file to be opened, use the following statements. The Open dialog box displays the files with the extension .bin only.
OpenFileDialog1.DefaultExt = ".bin"
OpenFileDialog1.AddExtension = True
OpenFileDialog1.Filter = "Binary Files|*.bin"
If OpenFileDialog1.ShowDialog() = _
Windows.Forms.DialogResult.OK Then
Debug.WriteLine(OpenFileDialog1.FileName)
End If
Code language: VB.NET (vbnet)
The following sections describe the properties of the OpenFileDialog and SaveFileDialog controls.
AddExtension
This property is a Boolean value that determines whether the dialog box automatically adds an extension to a filename if the user omits it. The extension added automatically is the one specified by the DefaultExtension property, which you must set before calling the ShowDialog method. This is the default extension of the files recognized by your application.
CheckFileExists
This property is a Boolean value that indicates whether the dialog box displays a warning if the user enters the name of a file that does not exist in the Open dialog box, or if the user enters the name of a file that exists in the Save dialog box.
CheckPathExists
This property is a Boolean value that indicates whether the dialog box displays a warning if the user specifies a path that does not exist, as part of the user-supplied filename.
DefaultExt
This property sets the default extension for the filenames specified on the control. Use this property to specify a default filename extension, such as .txt or .doc, so that when a file with no extension is specified by the user, the default extension is automatically appended to the filename. You must also set the AddExtension property to True. The default extension property starts with the period, and it’s a string — for example, .bin.
DereferenceLinks
This property indicates whether the dialog box returns the location of the file referenced by the shortcut or the location of the shortcut itself. If you attempt to select a shortcut on your desktop when the DereferenceLinks property is set to False, the dialog box will return to your application a value such as C:\WINDOWS\SYSTEM32\lnkstub.exe, which is the name of the shortcut, not the name of the file represented by the shortcut. If you set the DereferenceLinks property to True, the dialog box will return the actual filename represented by the shortcut, which you can use in your code.
FileName
Use this property to retrieve the full path of the file selected by the user in the control. If you set this property to a filename before opening the dialog box, this value will be the proposed filename. The user can click OK to select this file or select another one in the control. The two controls provide another related property, the FileNames property, which returns an array of filenames. To find out how to allow the user to select multiple files, see the discussion of the MultipleFiles and FileNames properties in ‘‘VB 2008 at Work: Multiple File Selection” at the end of this section.
Filter
This property is used to specify the type(s) of files displayed in the dialog box. To display text files only, set the Filter property to Text files|*.txt. The pipe symbol separates the description of the files (what the user sees) from the actual extension (how the operating system distinguishes the various file types).
If you want to display multiple extensions, such as .BMP, .GIF, and .JPG, use a semicolon to separate extensions with the Filter property. Set the Filter property to the string Images|*.BMP; *.GIF;*.JPG to display all the files of these three types when the user selects Images in the Save As Type combo box, under the box with the filename.
Don’t include spaces before or after the pipe symbol because these spaces will be displayed on the dialog box. In the Open dialog box of an image-processing application, you’ll probably provide options for each image file type, as well as an option for all images:
OpenFileDialog1.Filter = _
"Bitmaps|*.BMP|GIF Images|*.GIF|" & _
"JPEG Images|*.JPG|All Images|*.BMP;*.GIF;*.JPG"
Code language: VB.NET (vbnet)
FilterIndex
When you specify more than one file type when using the Filter property of the Open dialog box, the first file type becomes the default. If you want to use a file type other than the first one, use the FilterIndex property to determine which file type will be displayed as the default when the Open dialog box is opened. The index of the first type is 1, and there’s no reason to ever set this property to 1. If you use the Filter property value of the example in the preceding section and set the FilterIndex property to 2, the Open dialog box will display GIF files by default.
InitialDirectory
This property sets the initial folder whose files are displayed the first time that the Open and Save dialog boxes are opened. Use this property to display the files of the application’s folder or to specify a folder in which the application stores its files by default. If you don’t specify an initial folder, the dialog box will default to the last folder where the most recent file was opened or saved. It’s also customary to set the initial folder to the application’s path by using the following statement:
OpenFileDialog1.InitialDirectory = Application.ExecutablePath
Code language: VB.NET (vbnet)
The expression Application.ExecutablePath returns the path in which the application’s executable file resides.
RestoreDirectory
Every time the Open and Save As dialog boxes are displayed, the current folder is the one that was selected by the user the last time the control was displayed. The RestoreDirectory property is a Boolean value that indicates whether the dialog box restores the current directory before closing. Its default value is False, which means that the initial directory is not restored automatically. The InitialDirectory property overrides the RestoreDirectory property.
The following four properties are properties of the OpenFileDialog control only: FileNames, MultiSelect, ReadOnlyChecked, and ShowReadOnly.
FileNames
If the Open dialog box allows the selection of multiple files (see the later section “VB 2008 at Work: Multiple File Selection”), the FileNames property contains the pathnames of all selected files. FileNames is a collection, and you can iterate through the filenames with an enumerator. This property should be used only with the OpenFileDialog control, even though the SaveFileDialog control exposes a FileNames property.
MultiSelect
This property is a Boolean value that indicates whether the user can select multiple files in the dialog box. Its default value is False, and users can select a single file. When the MultiSelect property is True, the user can select multiple files, but they must all come from the same folder (you can’t allow the selection of multiple files from different folders). This property is unique to the OpenFileDialog control.
ReadOnlyChecked, ShowReadOnly
The ReadOnlyChecked property is a Boolean value that indicates whether the Read-Only check box is selected when the dialog box first pops up (the user can clear this box to open a file in read/write mode). You can set this property to True only if the ShowReadOnly property is also set to True. The ShowReadOnly property is also a Boolean value that indicates whether the Read-Only check box is available. If this check box appears on the form, the user can select it so the file will be opened as read-only. Files opened as read-only shouldn’t be saved onto the same file — always prompt the user for a new filename.
The OpenFile and SaveFile Methods
The OpenFileDialog control exposes the OpenFile method, which allows you to quickly open the selected file. Likewise, the SaveFileDialog control exposes the SaveFile method, which allows you to quickly save a document to the selected file. Normally, after retrieving the name of the file selected by the user, you must open this file for reading (in the case of the Open dialog box) or writing (in the case of the Save dialog box). The topic of reading from or writing to files is discussed in detail in Chapter, “Accessing Folders and Files.”
When this method is applied to the Open dialog box, the file is opened with read-only permission. The same method can be applied to the SaveFile dialog box, in which case the file is opened with read-write permission. Both methods return a Stream object, and you can call this object’s Read and Write methods to read from or write to the file.
OpenDialog and SaveDialog controls example: Multiple File Selection
The Open dialog box allows the selection of multiple files. This feature can come in handy when you want to process files en masse. You can let the user select many files, usually of the same type, and then process them one at a time. Or, you might want to prompt the user to select multiple files to be moved or copied.
To allow the user to select multiple files in the Open dialog box, set the MultiSelect property to True. The user can then select multiple files with the mouse by holding down the Shift or Ctrl key. The names of the selected files are reported by the property FileNames, which is an array of strings. The FileNames array contains the pathnames of all selected files, and you can iterate through them and process each file individually.
One of this chapter’s sample projects is the MultipleFiles project, which demonstrates how to use the FileNames property. The application’s form is shown in Figure 4.14. The button at the top of the form displays the Open dialog box, where you can select multiple files. After closing the dialog box by clicking the Open button, the application displays the pathnames of the selected files on a ListBox control.
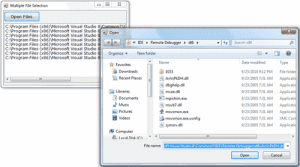
Figure 4.14 – Selecting multiple files in an open dialog box
The code behind the Open Files button is shown in Listing 4.17. In this example, I used the array’s enumerator to iterate through the elements of the FileNames array. You can use any of the methods discussed in the section “Arrays in Visual basic 2008” to iterate through the array.
Listing 4.17: Processing Multiple Selected Files
Private Sub bttnFile Click(...) _
Handles bttnFile.Click
OpenFileDialog1.Multiselect = True
OpenFileDialog1.ShowDialog()
Dim filesEnum As IEnumerator
ListBox1.Items.Clear()
filesEnum = OpenFileDialog1.FileNames.GetEnumerator()
While filesEnum.MoveNext
ListBox1.Items.Add(filesEnum.Current)
End While
End Sub
Code language: VB.NET (vbnet)