The ScrollBar and TrackBar controls let the user specify a magnitude by scrolling a selector between its minimum and maximum values. In some situations, the user doesn’t know in advance the exact value of the quantity to specify (in which case, a text box would suffice), so your application must provide a more-flexible mechanism for specifying a value, along with some type of visual feedback.
The vertical scroll bar that lets a user move up and down a long document is a typical example of the use of the ScrollBar control. The scroll bar and visual feedback are the prime mechanisms for repositioning the view in a long document or in a large picture thatwon’t fit entirely in its window.
The TrackBar control is similar to the ScrollBar control, but it doesn’t cover a continuous range of values. The TrackBar control has a fixed number of tick marks, which the developer can label. Users can place the slider’s indicator to he desired value.Whereas the ScrollBar control relies on some visual feedback outside the control to help the user position the indicator to the desired value, the TrackBar control forces the user to select from a range of valid values.
In short, the ScrollBar control should be used when the exact value isn’t as important as the value’s effect on another object or data element. The TrackBar control should be used when the user can type a numeric value and the value your application expects is a number in a specific range; for example, integers between 0 and 100, or a value between 0 and 5 inches in steps of 0.1 inches (0.0, 0.1, 0.2 . . . 5.0). The TrackBar control is preferred to the TextBox control in similar situations because there’s no need for data validation on your part. The user can specify only valid numeric values with the mouse.
The ScrollBar Control
There’s no ScrollBar control per se in the Toolbox; instead, there are two versions of it: the HScrollBar and VScrollBar controls. They differ only in their orientation, but because they share the same members, I will refer to both controls collectively as ScrollBar controls. Actually, both controls inherit from the ScrollBar control, which is an abstract control: It can be used to implement vertical and horizontal scroll bars, but it can’t be used directly on a form. Moreover, the HScrollBar and VScrollBar controls are not displayed in the Common Controls tab of the Toolbox. You have to open the All Windows Forms tab to locate these two controls.
The ScrollBar control is a long stripe with an indicator that lets the user select a value between the two ends of the control. The left (or bottom) end of the control corresponds to its minimum value; the other end is the control’s maximum value. The current value of the control is determined by the position of the indicator, which can be scrolled between the minimum and maximum values. The basic properties of the ScrollBar control, therefore, are properly named Minimum, Maximum, and Value.
- Minimum – The control’s minimum value. The default value is 0, but because this is an Integer value, you can set it to negative values as well.
- Maximum – The control’s maximum value. The default value is 100, but you can set it to any value that you can represent with the Integer data type.
- Value – The control’s current value, specified by the indicator’s position.
The Minimum and Maximum properties are Integer values. To cover a range of nonintegers, you must supply the code to map the actual values to Integer values. For example, to cover a range from 2.5 to 8.5, set the Minimum property to 25, set the Maximum property to 85, and divide the control’s value by 10. If the range you need is from –2.5 to 8.5, do the same but set the Minimum property to –25 and the Maximum value to 85, and divide the Value property by 10.
There are two more properties that allow you to control the movement of the indicator: the SmallChange and LargeChange properties. The first property is the amount by which the indicator changes when the user clicks one of the arrows at the two ends of the control. The LargeChange property is the displacement of the indicator when the user clicks somewhere in the scroll bar itself. You can manipulate a scroll bar by using the keyboard as well. Press the arrow keys to move the indicator in the corresponding direction by SmallChange, and the PageUp/PageDown keys to move the indicator by LargeChange.
The ScrollBar Control Colors Exercise
Figure 4.8 shows the main form of the Colors sample project, which lets the user specify a color by manipulating the value of its basic colors (red, green, and blue) through scroll bars. Each basic color is controlled by a scroll bar and has a minimum value of 0 and a maximum value of 255. If you aren’t familiar with color definition in the Windows environment, see the section “Specifying Colors” in Chapter, “Manipulating Images and Bitmaps.”
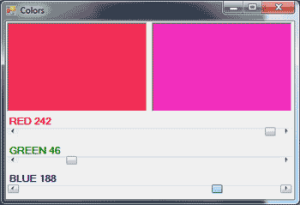
Figure 4.8 – When the ScrollBar is moved the corresponding color is displayed
As the scroll bar is moved, the corresponding color is displayed, and the user can easily specify a color without knowing the exact values of its primary components. All the user needs to know is whether the desired color contains, for example, too much red or too little green. With the help of the scroll bars and the immediate feedback from the application, the user can easily pinpoint the desired color. Notice that the exact values of the color’s basic components are of no practical interest; only the final color counts.
The ScrollBar Control’s Events
The user can change the ScrollBar control’s value in three ways: by clicking the two arrows at its ends, by clicking the area between the indicator and the arrows, and by dragging the indicator with the mouse. You can monitor the changes of the ScrollBar’s value from within your code by using two events: ValueChanged and Scroll. Both events are fired every time the indicator’s position is changed. If you change the control’s value from within your code, only the ValueChanged event will be fired.
The Scroll event can be fired in response to many different actions, such as the scrolling of the indicator with the mouse, a click on one of the two buttons at the ends of the scroll bars, and so on. If you want to know the action that caused this event, you can examine the Type property of the second argument of the event handler. The settings of the e.Type property are members of the ScrollEventType enumeration (LargeDecrement, SmallIncrement, Track, and so on).
Handling the Events in the Colors Application
The Colors application demonstrates how to program the two events of the ScrollBar control. The two PictureBox controls display the color designed with the three scroll bars. The left PictureBox is colored from within the Scroll event, whereas the other one is colored from within the ValueChanged event. Both events are fired as the user scrolls the scrollbar’s indicator, but in the Scroll event handler of the three scroll bars, the code examines the value of the e.Type property and reacts to it only if the event was fired because the scrolling of the indicator has ended. For all other actions, the event handler doesn’t update the color of the left PictureBox.
If the user attempts to change the Color value by clicking the two arrows of the scroll bars or by clicking in the area to the left or to the right of the indicator, both PictureBox controls are updated. While the user slides the indicator or keeps pressing one of the end arrows, only the PictureBox to the right is updated.
The conclusion from this experiment is that you can program either event to provide continuous feedback to the user. If this feedback requires too many calculations, which would slow down the reaction of the corresponding event handler, you can postpone the reaction until the user has stopped scrolling the indicator. You can detect this condition by examining the value of the e.Type property.When it’s ScrollEventType.EndScroll, you can execute the appropriate statements. Listing 6.16 shows the code behind the Scroll and ValueChanged events of the scroll bar that controls the red component of the color. The code of the corresponding events of the other two controls is identical.
Listing 4.16: Programming the ScrollBar Control’s Scroll Event
Private Sub redBar_Scroll(...) Handles redBar.Scroll
If e.Type = ScrollEventType.EndScroll Then
ColorBox1()
lblRed.Text = "RED " & redBar.Value.ToString("###")
End Sub
Private Sub redBar_ValueChanged(...) Handles redBar.ValueChanged
ColorBox2()
End Sub
Code language: VB.NET (vbnet)
The ColorBox1() and ColorBox2() subroutines update the color of the two PictureBox controls by setting their background colors. You can open the Colors project in Visual Studio and examine the code of these two routines.
The TrackBar Control
The TrackBar control is similar to the ScrollBar control, but it lacks the granularity of ScrollBar. Suppose that you want the user of an application to supply a value in a specific range, such as the speed of a moving object. Moreover, you don’t want to allow extreme precision; you need only a few settings, as shown in the examples in this page. The user can set the control’s value by sliding the indicator or by clicking on either side of the indicator.
Granularity is how specific youwant to be inmeasuring. Inmeasuring distances between towns, a granularity of a mile is quite adequate. In measuring (or specifying) the dimensions of a building, the granularity could be on the order of a foot or an inch. The TrackBar control lets you set the type of granularity that’s necessary for your application.
Similar to the ScrollBar control, SmallChange and LargeChange properties are available. SmallChange is the smallest increment by which the Slider value can change. The user can change the slider by the SmallChange value only by sliding the indicator. (Unlike the ScrollBar control, there are no arrows at the two ends of the Slider control.) To change the Slider’s value by LargeChange, the user can click on either side of the indicator.
The TrackBar Control Inches Exercise
Figure 4.9 demonstrates a typical use of the TrackBar control. The form in the figure is an element of a program’s user interface that lets the user specify a distance between 0 and 10 inches in increments of 0.2 inches. As the user slides the indicator, the current value is displayed on a Label control below the TrackBar. If you open the Inches application, you’ll notice that there are more stops than there are tick marks on the control. This is made possible with the TickFrequency property, which determines the frequency of the visible tick marks.
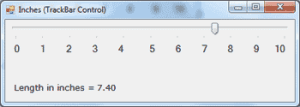
Figure 4.9 – A typical use of TrackBar control in VB.NET – The Inches Application
You might specify that the control has 50 stops (divisions), but that only 10 of them will be visible. The user can, however, position the indicator on any of the 40 invisible tick marks. You can think of the visible marks as the major tick marks, and the invisible ones as the minor tick marks. If the TickFrequency property is 5, only every fifth mark will be visible. The slider’s indicator, however, will stop at all tick marks.
When using the TrackBar control on your interfaces, you should set the TickFrequency property to a value that helps the user select the desired setting. Too many tick marks are confusing and difficult to read. Without tick marks, the control isn’t of much help. You might also consider placing a few labels to indicate the value of selected tick marks, as I have done in this example.
The properties of the TrackBar control in the Inches application are as follows:
Minimum = 0
Maximum = 50
SmallChange = 1
LargeChange = 5
TickFrequency = 5
Code language: VB.NET (vbnet)
The TrackBar needs to cover a range of 10 inches in increments of 0.2 inches. If you set the SmallChange property to 1, you have to set LargeChange to 5. Moreover, the TickFrequency is set to 5, so there will be a total of five divisions in every inch. The numbers below the tick marks were placed there with properly aligned Label controls.
The label at the bottom needs to be updated as the TrackBar’s value changes. This is signaled to the application with the Change event, which occurs every time the value of the control changes, either through scrolling or from within your code. The ValueChanged event handler of the TrackBar control is shown next:
Private Sub TrackBar1_ValueChanged(...) Handles TrackBar1.ValueChanged
lblInches.Text = "Length in inches = " & _
Format(TrackBar1.Value / 5, "#.00")
End Sub
Code language: VB.NET (vbnet)
The Label controls below the tick marks can also be used to set the value of the control. Every time you click one of the labels, the following statement sets the TrackBar control’s value. Notice that all the Label controls’ Click events are handled by a common handler:
Private Sub Label Click(...) _
Handles Label1.Click, Label9.Click
TrackBar1.Value = sender.text * 5
End Sub
Code language: VB.NET (vbnet)