Most practical applications are made up of multiple forms and dialog boxes, and one of the operations you’ll have to perform with multiform applications is to load and manipulate forms from within other forms’ code. For example, you might want to display a second form to prompt the user for data specific to an application. You must explicitly load the second form and read the information entered by the user when the auxiliary form is closed. Or you might want to maintain two forms open at once and let the user switch between them. A text editor and its Find & Replace dialog box is a typical example (e.g.:- Notepad, Wordpad).
You can access a form from within another form by its name. Let’s say that your application has two forms, named Form1 and Form2, and that Form1 is the project’s startup form. To show Form2 when an action takes place on Form1, call the Show method of the auxiliary form:
Form2.Show
Code language: VB.NET (vbnet)
This statement brings up Form2 and usually appears in a button’s or menu item’s Click event handler. To exchange information between two forms, use the techniques described in the “Controlling One Form from within Another,” section later in this chapter.
The Show method opens a form in a modeless manner: The two forms are equal in stature on the desktop, and the user can switch between them. You can also display the second form in a modal manner, which means that users can’t return to the form from which they invoked it without closing the second form. While a modal form is open, it remains on top of the desktop, and you can’t move the focus to any other form of the same application (but you can switch to another application). To open a modal form, use the ShowDialog method:
Form2.ShowDialog
Code language: VB.NET (vbnet)
The modal form is, in effect, a dialog box like the Open File dialog box. You must first select a file on this form and click the Open button, or click the Cancel button to close the dialog box and return to the form from which the dialog box was invoked. This brings up the topic of forms and dialog boxes.
A dialog box is simply a modal form. When we display forms as dialog boxes, we change the border of the forms to the setting FixedDialog and invoke them with the ShowDialog method. Modeless forms are more difficult to program because the user may switch among them at any time. Moreover, the two forms that are open at once must interact with one another. When the user acts on one of the forms, it might necessitate some changes in the other, and you’ll see shortly how this is done. If the two active forms don’t need to interact, display one of them as a dialog box.
When you’re finished with the second form, you can either close it by calling its Close method or hide it by calling its Hide method. The Close method closes the form, and its resources are returned to the system. The Hide method sets the form’s Visible property to False; you can still access a hidden form’s controls from within your code, but the user can’t interact with it. Forms that are displayed often, such as the Find & Replace dialog box of a text-processing application, should be hidden — not closed. To the user, it makes no difference whether you hide or close a form. If you hide a form, however, the next time you bring it up with the Show method, its controls are in the state they were the last time.
The Startup Form
A typical application has more than a single form. When an application starts, the main form is loaded. You can control which form is initially loaded by setting the startup object in the project Properties window, shown in Figure 5.10. To open this dialog box, right-click the project’s name in the Solution Explorer and select Properties. In the project’s Properties pages, select the Application tab and select the appropriate item in the Startup Form combo box.
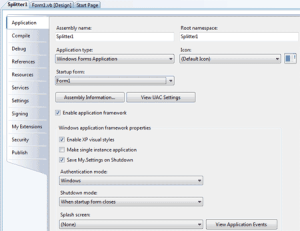
Figure 5.10 – In the Project properties specifying the start up Form that’s displayed when application starts
By default, the IDE suggests the name of the first form it created, which is Form1. If you change the name of the form, Visual Basic will continue using the same form as the startup form with its new name.
You can also start an application by using a subroutine, without loading a form. This subroutine is the MyApplication Startup event handler, which is fired automatically when the application starts. To display the AuxiliaryForm object from within the Startup event handler, use the following statement:
Private Sub MyApplication_Startup(...) Handles Me.Startup
System.Windows.Forms.Application.Run(New AuxiliaryForm())
End Sub
Code language: VB.NET (vbnet)
To view the MyApplication Startup event handler, click the View Application Events button at the bottom of the Application pane, as shown in Figure 5.10. This action will take you to the MyApplication code window, where you can select the MyApplication Events item in the object list and the Startup item in the events list.