Although the ComboBox control allows users to enter text in the control’s edit box, it doesn’t provide a simple mechanism for adding new items at runtime. Let’s say you provide a ComboBox with city names. Users can type the first few characters and quickly locate the desired item. But what if you want to allow users to add new city names? You can provide this feature with two simple techniques. The simpler one is to place a button with an ellipsis (three periods) right next to the control. When users want to add a new item to the control, they can click the button and be prompted for the new item.
A more-elegant approach is to examine the control’s Text property as soon as the control loses focus, or the user presses the Enter key. If the string entered by the user doesn’t match an item on the control, you must add a new item to the control’s Items collection and select the new itemfrom within your code. The FlexComboBox project demonstrates how to use both techniques in your code. The main form of the project, which is shown in Figure 4.7, is a simple data-entry screen. It’s not the best data-entry form, but it’s meant for demonstration purposes.
You can either enter a city name (or country name) and press the Tab key to move to another control or click the button next to the control to be prompted for a new city/country name. The application will let you enter any city/country combination. You should provide code to limit the cities within the selected country, but this is a nontrivial task. You also need to store the new city names entered on the first ComboBox control to a file (or a database table), so users will find them there the next time they execute the application. I haven’t made the application elaborate; I’ve added the code only to demonstrate how to add new items to a ComboBox control at runtime.
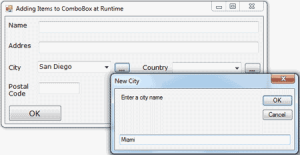
Figure 4.7 – Adding items to ComboBox control at runtime
VB.NET ComboBox Control Example
The ellipsis button next to the City ComboBox control prompts the user for the new item via the InputBox() function. Then it searches the Items collection of the control via the FindString method, and if the new item isn’t found, it’s added to the control. Then the code selects the new item in the list. To do so, it sets the control’s SelectedIndex property to the value returned by the Items.Add method, or the value returned by the FindString method, depending on whether the item was located or added to the list. Listing 4.14 shows the code behind the ellipsis button.
Listing 4.14: Adding a New Item to the ComboBox Control at Runtime
Private Sub bttnLocateCity_Click(...) Handles bttnLocateCity.Click
Dim itm As String
itm = InputBox("Enter a city name", "New City")
If itm <> "" Then
AddElement(cbCity, itm)
End If
End Sub
Code language: VB.NET (vbnet)
The AddElement() subroutine, which accepts a string as an argument and adds it to the control, is shown in Listing 4.15. If the item doesn’t exist in the control, it’s added to the Items collection. If the item is a member of the Items collection, it’s selected. As you will see, the same subroutine will be used by the second method for adding items to the control at runtime.
Listing 4.15: The AddElement() Subroutine
Sub AddElement(ByRef control As ComboBox, ByVal newItem As String)
Dim idx As Integer
If control.FindString(newItem) >= 0 Then
idx = control.FindString(newItem)
Else
idx = control.Items.Add(newItem)
End If
control.SelectedIndex = idx
End Sub
Code language: VB.NET (vbnet)
You can also add new items at runtime by adding the same code in the control’s LostFocus event handler:
Private Sub ComboBox1 LostFocus(...) Handles ComboBox1.LostFocus
Dim newItem As String = ComboBox1.Text
AddElement(newItem)
End Sub
Code language: VB.NET (vbnet)