As with the TreeView control, the ListView control can be populated either at design time or at runtime. To add items at design time, click the ellipsis button next to the ListItems property in the Properties window. When the ListViewItem Collection Editor dialog box pops up, you can enter the items, including their subitems, as shown in Figure 4.30.
Click the Add button to add a new item. Each item has subitems, which you can specify as members of the SubItems collection. To add an item with three subitems, you must populate the item’s SubItems collection with the appropriate elements. Click the ellipsis button next to the SubItems property in the ListViewItem Collection Editor; the ListViewSubItem Collection Editor will appear. This dialog box is similar to the ListViewItem Collection Editor dialog box, and you can add each item’s subitems. Assuming that you have added the item called Item 1 in the ListViewItem Collection Editor, you can add these subitems: Item 1-a, Item 1-b, and Item 1-c. The first subitem (the one with zero index) is actually the main item of the control.
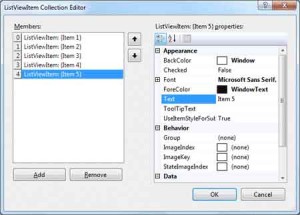
Figure 4.30 – ListViewItem Collection Editor Dialog Box
Notice that you can set other properties such as the color and font for each item, the check box in front of the item that indicates whether the item is selected, and the image of the item. Use this window to experiment with the appearance of the control and the placement of the items, especially in Details view because subitems are visible only in this view. Even then, you won’t see anything unless you specify headers for the columns. Note that you can add more subitems than there are columns in the control. Some of the subitems will remain invisible.
Unlike the TreeView control, the ListView control allows you to specify a different appearance for each item and each subitem. To set the appearance of the items, use the Font, BackColor, and ForeColor properties of the ListViewItem object.
Almost all ListView controls are populated at runtime. Not only that, but you should be able to add and remove items during the course of the application. The items of the ListView control are of the ListViewItem type, and they expose members that allow you to control the appearance of the items on the control. These members are as follows:
BackColor/ForeColor properties – These properties set or return the background/foreground colors of the current item or subitem.
Checked property – This property controls the status of an item. If it’s True, the item has been selected. You can also select an item from within your code by setting its Checked property to True. The check boxes in front of each item won’t be visible unless you set the control’s ShowCheckBoxes property to True.
Font property – This property sets the font of the current item. Subitems can be displayed in a different font if you specify one by using the Font property of the corresponding subitem (see the section titled ‘‘The SubItems Collection,” later in this chapter). By default, subitems inherit the style of the basic item. To use a different style for the subitems, set the item’s UseItemStyleForSubItems property to False.
Text property – This property indicates the caption of the current item or subitem.
SubItems collection – This property holds the subitems of a ListViewItem. To retrieve a specific subitem, use a statement such as the following:
sitem = ListView1.Items(idx1).SubItems(idx2)
Code language: VB.NET (vbnet)
where idx1 is the index of the item, and idx2 is the index of the desired subitem.*
To add a new subitem to the SubItems collection, use the Add method, passing the text of the subitem as an argument:
LItem.SubItems.Add("subitem's caption")
Code language: VB.NET (vbnet)
The argument of the Add method can also be a ListViewItem object. Create a ListViewItem, populate it, and then add it to the Items collection as shown here:
Dim LI As New ListViewItem
LI.Text = "A New Item"
Li.SubItems.Add("Its first subitem")
Li.SubItems.Add("Its second subitem")
' statements to add more subitems
ListView1.Items.Add(LI)
Code language: VB.NET (vbnet)
If you want to add a subitem at a specific location, use the Insert method. The Insert method of the SubItems collection accepts two arguments: the index of the subitem before which the new subitem will be inserted, and a string or ListViewItem to be inserted:
LItem.SubItems.Insert(idx, subitem)
Code language: VB.NET (vbnet)
Like the ListViewItem objects, each subitem can have its own font, which is set with the Font property.
The items of the ListView control can be accessed through the Items property, which is a collection. As such, it exposes the standard members of a collection, which are described in the following section. Its item has a SubItems collection that contains all the subitems of the corresponding item.