Sometimes we need to prompt users for a folder, rather than a filename. An application that processes files in batch mode shouldn’t force users to select the files to be processed. Instead, it should allow users to select a folder and process all files of a specific type in the folder (it could encrypt all text documents or resize all image files, for example). As elaborate as the File Open dialog box might be, it doesn’t allow the selection of a folder. To prompt users for a folder’s path, use the FolderBrowser dialog box, which is a very simple one; it’s shown in Figure 4.15 in “Folder Browsing Example Project.” listed below. The FolderBrowserDialog control exposes a small number of properties, which are discussed next.
RootFolder
This property indicates the initial folder to be displayed when the dialog box is shown. It is not necessarily a string; it can also be a member of the SpecialFolder enumeration. To see the members of the enumeration, enter the following expression:
FolderBrowserDialog1.RootFolder =
Code language: VB.NET (vbnet)
As soon as you enter the equals sign, you will see the members of the enumeration. The most common setting for this property is My Computer, which represents the target computer’s file system. You can set the RootFolder property to a number of special folders (for example, Personal, Desktop, ApplicationData, LocalApplicationData, and so on). You can also set this property to a string with the desired folder’s pathname.
SelectedFolder
After the user closes the FolderBrowser dialog box by clicking the OK button, you can retrieve the name of the selected folder with the SelectedFolder property, which is a string, and you can use it with the methods of the System.IO namespace to access and manipulate the selected folder’s files and subfolders.
ShowNewFolderButton
This property determines whether the dialog box will contain a New button; its default value is True. When users click the New button to create a new folder, the dialog box prompts them for the new folder’s name, and creates a new folder with the specified name under the selected folder.
Folder Browsing Example Project – VB.NET 2008
The FolderBrowser control is a trivial control, but I’m including a sample application to demonstrate its use. The same application demonstrates how to retrieve the files and subfolders of the selected folder and how to create a directory listing in a RichTextBox control, like the one shown in Figure 4.15. The members of the System.IO namespace, which allow you to access and manipulate files and folders from within your code, are discussed in detail in Chapter “Accessing Folders and Files”.
The FolderBrowser dialog box is set to display the entire file system of the target computer and is invoked with the following statements:
FolderBrowserDialog1.RootFolder = Environment.SpecialFolder.MyComputer
FolderBrowserDialog1.ShowNewFolderButton = False
If FolderBrowserDialog1.ShowDialog = DialogResult.OK Then
’ process files in selected folder
End If
Code language: VB.NET (vbnet)
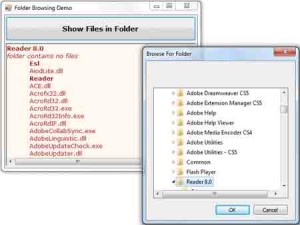
Figure 4.15 – Selecting a folder via the FolderBrowser dialog box
As usual, we examine the value returned by the ShowDialog method of the control and we proceed if the user has closed the dialog box by clicking the OK button. The code that iterates through the selected folder’s files and subfolders, shown in Listing 4.18, is basically a demonstration of some members of the System.IO namespace, but I’ll review it briefly here.
Listing 4.18: Scanning a Folder
Private Sub bttnSelectFiles_Click(...) _
Handles bttnSelectFiles.Click
FolderBrowserDialog1.RootFolder = _
Environment.SpecialFolder.MyComputer
FolderBrowserDialog1.ShowNewFolderButton = False
If FolderBrowserDialog1.ShowDialog = Windows.Forms.DialogResult.OK Then
RichTextBox1.Clear()
Me.Cursor = Cursors.WaitCursor
Application.DoEvents()
' Retrieve initial folder
Dim initialFolder As String = _
FolderBrowserDialog1.SelectedPath
Dim InitialFolder As New IO.DirectoryInfo( _
FolderBrowserDialog1.SelectedPath)
' and print its name (no indentation for top item)
PrintFolderName(InitialFolder, "")
' and then print the files in the top folder
If InitialFolder.GetFiles("*.*").Length = 0 Then
SwitchToItalics()
RichTextBox1.AppendText( _
"folder contains no files" & vbCrLf)
SwitchToRegular()
Else
PrintFileNames(InitialFolder, "")
End If
Dim DI As IO.DirectoryInfo
' Iterate through every subfolder and print it
For Each DI In InitialFolder.GetDirectories
PrintDirectory(DI)
Next
Me.Cursor = Cursors.Default
End If
End Sub
Code language: VB.NET (vbnet)
The selected folder’s name is stored in the initialFolder variable and is passed as an argument to the constructor of the DirectoryInfo class. The InitialDir variable represents the specified folder. This object is passed to the PrintFolderName() subroutine, which prints the folder’s name in bold. Then the code iterates through the same folder’s files and prints them with the PrintFileNames() subroutine, which accepts as an argument the DirectoryInfo object that represents the current folder and the indentation level. After printing the initial folder’s name and the names of the files in the folder, the code iterates through the subfolders of the initial folder. The GetDirectories method of the DirectoryInfo class returns a collection of objects, one for each subfolder under the folder represented by the InitialDir variable. For each subfolder, it calls the PrintDirectory() subroutine, which prints the folder’s name and the files in this folder, and then iterates through the folder’s subfolders. The code that iterates through the selected folder’s files and subfolders is shown in Listing 4.19.
Listing 4.19: The PrintDirectory() Subroutine
Private Sub PrintDirectory(ByVal CurrentDir As IO.DirectoryInfo)
Static IndentationLevel As Integer = 0
IndentationLevel += 1
Dim indentationString As String = ””
indentationString = _
New String(Convert.ToChar(vbTab), IndentationLevel)
PrintFolderName(CurrentDir, indentationString)
If CurrentDir.GetFiles(”*.*”).Length = 0 Then
SwitchToItalics()
RichTextBox1.AppendText(indentationString & _
”folder contains no files” & vbCrLf)
SwitchToRegular()
Else
PrintFileNames(CurrentDir, indentationString)
End If
Dim folder As IO.DirectoryInfo
For Each folder In CurrentDir.GetDirectories
PrintDirectory(folder)
Next
IndentationLevel -= 1
End Sub
Code language: VB.NET (vbnet)
The code that iterates through the subfolders of a given folder is discussed in detail in Chapter “Accessing Folders and Files”, so you don’t have to worry if you can’t figure out how it works yet. In the next section, you’ll learn how to display formatted text in the RichTextBox control.