The ListView control is similar to the ListBox control except that it can display its items in many forms, along with any number of subitems for each item. To use the ListView control in your project, place an instance of the control on a form and then set its basic properties, which are described in the following list.
View and Arrange – Two properties determine how the various items will be displayed on the control: the View property, which determines the general appearance of the items, and the Arrange property, which determines the alignment of the items on the control’s surface. The View property can have one of the values shown in Table 4.8.
Table 4.8: Settings of the View Property of VB.NET ListView Control
Setting | Description |
---|---|
LargeIcon | (Default) Each item is represented by an icon and a caption below the icon. |
SmallIcon | Each item is represented by a small icon and a caption that appears to the right of the icon. |
List | Each item is represented by a caption. |
Details | Each item is displayed in a column with its subitems in adjacent columns. |
Tile | Each item is displayed with an icon and its subitems to the right of the icon. This view is available only on Windows XP and Windows Server 2003. |
The Arrange property can have one of the settings shown in Table 4.9.
Table 4.9: Settings of the Arrange Property of VB.NET ListView Control
Setting | Description |
---|---|
Default | When an item is moved on the control, the item remains where it is dropped. |
Left | Items are aligned to the left side of the control. |
SnapToGrid | Items are aligned to an invisible grid on the control. When the user moves an item, the item moves to the closest grid point on the control. |
Top | Items are aligned to the top of the control. |
HeaderStyle – This property determines the style of the headers in Details view. It has no meaning when the View property is set to anything else, because only the Details view has columns. The possible settings of the HeaderStyle property are shown in Table 4.10.
Table 4.10: Settings of the HeaderStyle Property of VB.NET ListView Control
Setting | Description |
---|---|
Clickable | Visible column header that responds to clicking |
Nonclickable | (Default) Visible column header that does not respond to clicking |
None | No visible column header |
AllowColumnReorder – This property is a True/False value that determines whether the user can reorder the columns at runtime, and it’s meaningful only in Details view. If this property is set to True, the user can move a column to a new location by dragging its header with the mouse and dropping it in the place of another column.
Activation – This property, which specifies how items are activated with the mouse, can have one of the values shown in Table 4.11.
Table 4.11: Settings of the Activation Property of VB.NET ListView Control
Setting | Description |
---|---|
OneClick | Items are activated with a single click. When the cursor is over an item, it changes shape, and the color of the item’s text changes. |
Standard | (Default) Items are activated with a double-click. No change in the selected item’s text color takes place. |
TwoClick | Items are activated with a double-click, and their text changes color as well. |
FullRowSelect – This property is a True/False value, indicating whether the user can select an entire row or just the item’s text, and it’s meaningful only in Details view. When this property is False, only the first item in the selected row is highlighted.
GridLines – Another True/False property. If True, grid lines between items and subitems are drawn. This property is meaningful only in Details view.
Group – The items of the ListView control can be grouped into categories. To use this feature, you must first define the groups by using the control’s Group property, which is a collection of strings. You can add as many members to this collection as you want. After that, as you add items to the ListView control, you can specify the group to which they belong. The control will group the items of the same category together and display the group’s title above each group. You can easily move items between groups at runtime by setting the corresponding item’s Group property to the name of the desired group.
LabelEdit – The LabelEdit property lets you specify whether the user will be allowed to edit the text of the items. The default value of this property is False. Notice that the LabelEdit property applies to the item’s Text property only; you can’t edit the subitems (unfortunately, you can’t use the ListView control as an editable grid).
MultiSelect – A True/False value, indicating whether the user can select multiple items from the control. To select multiple items, click them with the mouse while holding down the Shift or Ctrl key. If the control’s ShowCheckboxes property is set to True, users can select multiple items by marking the check box in front of the corresponding item(s).
Scrollable – A True/False value that determines whether the scroll bars are visible. Even if the scroll bars are invisible, users can still bring any item into view. All they have to do is select an item and then press the arrow keys as many times as needed to scroll the desired item into view.
Sorting – This property determines how the items will be sorted, and its setting can be None, Ascending, or Descending. To sort the items of the control, call the Sort method, which sorts the items according to their caption. It’s also possible to sort the items according to any of their subitems, as explained in the section “Sorting the ListView Control” later in this chapter.
The Columns Collection of ListView Control in VB.NET 2008
To display items in Details view, you must first set up the appropriate columns. The first column corresponds to the item’s caption, and the following columns correspond to its subitems. If you don’t set up at least one column, no items will be displayed in Details view. Conversely, the Columns collection is meaningful only when the ListView control is used in Details view.
The items of the Columns collection are of the ColumnHeader type. The simplest way to set up the appropriate columns is to do so at design time by using a visual tool. Locate and select the Columns property in the Properties window, and click the ellipsis button next to the property. The ColumnHeader Collection Editor dialog box will appear, as shown in Figure 4.29, in which you can add and edit the appropriate columns.
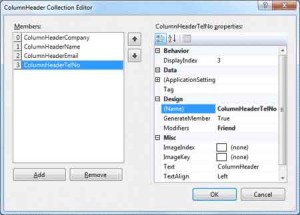
Figure 4.29 – ListView Control’s Column Header Collection Editor Dialog Box
Adding columns to a ListView control and setting their properties through the dialog box shown in Figure 4.29 is quite simple. Don’t forget to size the columns according to the data you anticipate storing in them and to set their headers.
It is also possible to manipulate the Columns collection from within your code as follows. Create a ColumnHeader object for each column in your code, set its properties, and then add it to the control’s Columns collection:
Dim ListViewCol As New ColumnHeader
ListViewCol.Text = "New Column"
ListViewCol.TextAlign = HorizontalAlignment.Center
ListViewCol.Width = 125
ListView1.Columns.Add(ListViewCol)
Code language: VB.NET (vbnet)
Adding and Removing Columns at Runtime
To add a new column to the control, use the Add method of the Columns collection. The syntax of the Add method is as follows:
ListView1.Columns.Add(header, width, textAlign)
Code language: VB.NET (vbnet)
The header argument is the column’s header (the string that appears on top of the items). The width argument is the column’s width in pixels, and the last argument determines how the text will be aligned. The textAlign argument can be Center, Left, or Right.
The Add method returns a ColumnHeader object, which you can use later in your code to manipulate the corresponding column. The ColumnHeader object exposes a Name property, which can’t be set with the Add method:
Header1 = TreeView1.Add( _
"Column 1", 60, ColAlignment.Left)
Header1.Name = "Column1"
Code language: VB.NET (vbnet)
After the execution of these statements, the first column can be accessed not only by index, but also by name.
To remove a column, call the Remove method:
ListView1.Columns(3).Remove
Code language: VB.NET (vbnet)
The indices of the following columns are automatically decreased by one. The Clear method removes all columns from the Columns collection. Like all collections, the Columns collection exposes the Count property, which returns the number of columns in the control.