Let’s put together the members of the ListView control to create a sample application that populates the control and enumerates its items. The sample application of this section is the ListViewDemo project. The application’s form, shown in Figure 4.31, contains a ListView control whose items can be displayed in all possible views, depending on the status of the RadioButton controls in the List Style section on the right side of the form.
The control’s headers and their widths were set at design time through the ColumnHeader Collection Editor, as explained earlier. To populate the ListView control, click the Populate List button, whose code is shown next. The code creates a new ListViewItem object for each item to be added. Then it calls the Add method of the SubItems collection to add the item’s subitems (contact, phone, and fax numbers). After the ListViewItem has been set up, it’s added to the control via the Add method of its Items collection.
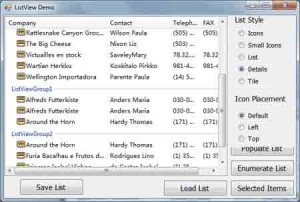
Figure 4.31 – ListView Sample Project demonstrates the basic members of the ListView control.
Listing 4.46 shows the statements that insert the first two items in the list. The remaining items are added by using similar statements, which need not be repeated here. The sample data I used in the ListViewDemo application came from the Northwind sample database.
Listing 4.46: Populating a ListView Control
Dim LItem As New ListViewItem()
LItem.Text = "Alfred's Futterkiste"
LItem.SubItems.Add("Anders Maria")
LItem.SubItems.Add("030-0074321")
LItem.SubItems.Add("030-0076545")
LItem.ImageIndex = 0
ListView1.Items.Add(LItem)
LItem = New ListViewItem()
LItem.Text = "Around the Horn"
LItem.SubItems.Add("Hardy Thomas")
LItem.SubItems.Add("(171) 555-7788")
LItem.SubItems.Add("(171) 555-6750")
LItem.ImageIndex = 0
ListView1.Items.Add(LItem)
Code language: VB.NET (vbnet)
Enumerating the List
The Enumerate List button scans all the items in the list and displays them along with their subitems in the Immediate window. To scan the list, you must set up a loop that enumerates all the items in the Items collection. For each item in the list, set up a nested loop that scans all the subitems of the current item. The complete code for the Enumerate List button is shown in Listing 4.47.
Listing 4.47: Enumerating Items and SubItems
Private Sub bttnEnumerate Click(...) _
Handles bttnEnumerate.Click
Dim i, j As Integer
Dim LItem As ListViewItem
For i = 0 To ListView1.Items.Count - 1
LItem = ListView1.Items(i)
Debug.WriteLine(LItem.Text)
For j = 0 To LItem.SubItems.Count - 1
Debug.WriteLine(" " & ListView1.Columns(j).Text & _
" " & Litem.SubItems(j).Text)
Next
Next
End Sub
Code language: VB.NET (vbnet)
Notice that each item may have a different number of subitems. The output of this code in the Immediate window is shown next. The subitems appear under the corresponding item, and they are indented by three spaces:
Alfred's Futterkiste
Company Alfred's Futterkiste
Contact Anders Maria
Telephone 030-0074321
FAX 030-0076545
Around the Horn
Company Around the Horn
Contact Hardy Thomas
Telephone (171) 555-7788
FAX (171) 555-6750
Code language: VB.NET (vbnet)
The code in Listing 4.47 uses a For. . .Next loop to iterate through the items of the control. You can also set up a For Each. . .Next loop, as shown here:
Dim LI As ListViewItem
For Each LI In ListView1.Items
{ access the current item through the LI variable}
Next
Code language: VB.NET (vbnet)