The CustomExplorer application, which demonstrates the basic properties and methods of the Directory and File classes, duplicates the functionality of Windows Explorer. Its user interface, shown in Figure 11.1, was discussed in section, “The TreeView Control.” In this chapter, you’ll see how to access the file system and populate the two controls with folder names and filenames, using the basic members of the Directory and File classes of the System.IO namespace. You can implement the same application with the FileSystem component as an exercise. I will post the same application implemented with the FileSystem component of the My object in this chapter’s Projects folder for your convenience.
When you start the application, the names of the subfolders under the C:\Program Files folder are displayed in the TreeView control, in a hierarchical structure. This operation takes a few seconds because the code must scan the entire folder, including its subfolders, and generate the necessary nodes on the TreeView control. The more programs you have installed on your C: drive, the longer it will take to scan them. Change the value of the initFolder variable in Listing 11.7 to scan a different folder. As the code iterates through the subfolders, it displays the name of the current folder on the form’s title bar, so that you can monitor the progress of the operation. This is one form of feedback you can provide for this operation.
Listing 11.7: CustomExplorer’s Form Load Event Handler
Private Sub Form1_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
Dim Nd As New TreeNode
Dim initFolder As String = "C:\Program Files"
Nd = TreeView1.Nodes.Add(initFolder)
Me.Show()
Application.DoEvents()
Me.Cursor = Cursors.WaitCursor
ScanFolder(initFolder, Nd)
Me.Cursor = Cursors.Default
End Sub
Code language: PHP (php)
As you can guess, all the work is done by the ScanFolder() subroutine, which accepts as arguments the path of the folder to scan and the current node on the TreeView control. The subfolders of the specified folder will be added to the TreeView control as child nodes of the current node, and this is why the ScanFolder() subroutine needs a reference to the current node.
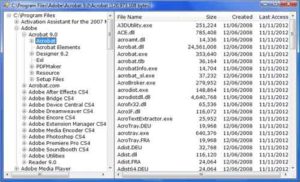
The ScanFolder() subroutine iterates through the subfolders of the specified folder recursively and creates a new node for each subfolder. If a subfolder contains subfolders of its own, the ScanFolder() subroutine calls itself, passing the name of the subfolder as an argument. This way, each folder is scanned completely, regardless of it depth (the levels of nested subfolders). Listing 11.8 shows the code of the ScanFolders() subroutine.
Listing 11.8: Displaying the Subfolders of the Selected Folder
Sub ScanFolder(ByVal folderSpec As String, ByRef currentNode As TreeNode)
Dim thisFolder As String
Dim allFolders() As String
allFolders = IO.Directory.GetDirectories(folderSpec)
For Each thisFolder In allFolders
Dim Nd As TreeNode
Nd = New TreeNode(Path.GetFileName(thisFolder))
currentNode.Nodes.Add(Nd)
folderSpec = thisFolder
ScanFolder(folderSpec, Nd)
Me.Text = "Scanning " & folderSpec
Me.Refresh()
Next
End Sub
Code language: PHP (php)
The ScanFolder() subroutine is surprisingly simple because it’s recursive: It calls itself again and again to iterate through all the subfolders of the specified folder. The GetDirectories method retrieves the names of the subfolders of the current folder and returns them as an array of strings: the allFolders array. The following loop iterates through the elements of this array. For each element, it adds a new node under the current node on the TreeView control and then calls itself, passing the current folder’s name as an argument. If the current folder contains subfolders, they will be added to the TreeView control as well.
In the Form’s Load event handler, we populate the TreeView control with the hierarchy of the initial folder’s subfolders. You should probably provide a Browse For Folder dialog box to allow users to select the folder (or drive) to be mapped on the TreeView control. To view the files in a specific folder, you can click the folder’s name in the TreeView control. The ListView control on the left will be populated with the names and basic properties of the files in the selected folder. The code that displays the list of files in the selected folder resides in the AfterSelect event handler of the TreeView control (it’s shown in Listing 11.9).
Listing 11.9: Displaying a Folder’s Files
Private Sub TreeView1_AfterSelect(ByVal sender As System.Object, _
ByVal e As System.Windows.Forms.TreeViewEventArgs) _
Handles TreeView1.AfterSelect
Dim Nd As TreeNode
Dim pathName As String
Nd = TreeView1.SelectedNode
pathName = Nd.FullPath
Me.Text = pathName
ShowFiles(pathName)
End Sub
Code language: PHP (php)
The ShowFiles() subroutine accepts as an argument a folder path and displays the files in the folder, along with their basic properties. Its code, which is shown in Listing 11.10, iterates through the array with the filenames returned by the Directory.GetFiles method and uses the FileInfo class to retrieve each file’s basic properties.
Listing 11.10: ShowFiles() Subroutine
Sub ShowFiles(ByVal selFolder As String)
ListView1.Items.Clear()
Dim files() As String
Dim file As String
files = IO.Directory.GetFiles(selFolder)
Dim TotalSize As Long
Dim FI As IO.FileInfo
For Each file In files
Dim LItem As New ListViewItem
LItem.Text = IO.Path.GetFileName(file)
FI = New IO.FileInfo(file)
LItem.SubItems.Add(FI.Length.ToString("#,###"))
LItem.SubItems.Add(FI.CreationTime.ToShortDateString)
LItem.SubItems.Add(FI.LastAccessTime.ToShortDateString)
ListView1.Items.Add(LItem)
TotalSize += FI.Length
Next
Me.Text = Me.Text & " [" & TotalSize.ToString("#,###") & " bytes]"
End Sub
Code language: PHP (php)