One-dimensional arrays, such as those presented so far, are good for storing long sequences of one-dimensional data (such as names or temperatures). But how would you store a list of cities and their average temperatures in an array? Or names and scores; years and profits; or data with more than two dimensions, such as products, prices, and units in stock? In some situations, you will want to store sequences of multidimensional data. You can store the same data more conveniently in an array of as many dimensions as needed.
Figure 2.5 shows two one-dimensional arrays — one of them with city names, the other with temperatures. The name of the third city would be City(2), and its temperature would be Temperature(2).
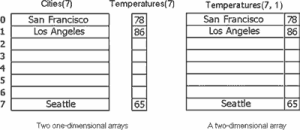
Figure 2.5 – Two one-dimensional arrays and the equivalent two-dimensional array
A two-dimensional array has two indices: The first identifies the row (the order of the city in the array), and the second identifies the column (city or temperature). To access the name and temperature of the third city in the two-dimensional array, use the following indices:
Temperatures(2, 0) ' is the third city's name
Temperatures(2, 1) ' is the third city's average temperature
Code language: VB.NET (vbnet)
The benefit of using multidimensional arrays is that they’re conceptually easier to manage. Suppose that you’re writing a game and want to track the positions of certain pieces on a board. Each square on the board is identified by two numbers: its horizontal and vertical coordinates. The obvious structure for tracking the board’s squares is a two-dimensional array, in which the first index corresponds to the row number, and the second corresponds to the column number.
The array could be declared as follows:
Dim Board(9, 9) As Integer
Code language: VB.NET (vbnet)
When a piece is moved from the square in the first row and first column to the square in the third row and fifth column, you assign the value 0 to the element that corresponds to the initial position:
Board(0, 0) = 0
Code language: VB.NET (vbnet)
And you assign 1 to the square to which it was moved to indicate the new state of the board:
Board(2, 4) = 1
Code language: VB.NET (vbnet)
To find out whether a piece is on the top-left square, you’d use the following statement:
If Board(0, 0) = 1 Then
{ piece found}
Else
{ empty square}
End If
Code language: VB.NET (vbnet)
This notation can be extended to more than two dimensions. The following statement creates an array with 1,000 elements (10 by 10 by 10):
Dim Matrix(9, 9, 9)
Code language: VB.NET (vbnet)
You can think of a three-dimensional array as a cube made up of overlaid two-dimensional arrays, such as the one shown in Figure 2.6.
Figure 2.6 – Pictorial representations of one-, two-, and three-dimensional arrays
It is possible to initialize a multidimensional array with a single statement, just as you do with a one-dimensional array. You must insert enough commas in the parentheses following the array name to indicate the array’s rank. The following statements initialize a two-dimensional array and then print a couple of its elements:
Dim a(,) As Integer = {{10, 20, 30}, {11, 21, 31}, {12, 22, 32}}
Console.WriteLine(a(0, 1)) ' will print 20
Console.WriteLine(a(2, 2)) ' will print 32
Code language: VB.NET (vbnet)
You should break the line that initializes the dimensions of the array into multiple lines to make your code easier to read. Just insert the line continuation character at the end of each continued line:
Dim a(,) As Integer = {{10, 20, 30},
{11, 21, 31},
{12, 22, 32}}
Code language: VB.NET (vbnet)
If the array has more than one dimension, you can find out the number of dimensions with the Array.Rank property. Let’s say you have declared an array for storing names and salaries by using the following statements:
Dim Employees(1,99) As Employee
Code language: VB.NET (vbnet)
To find out the number of dimensions, use the following statement:
Employees.Rank
Code language: VB.NET (vbnet)
When using the Length property to find out the number of elements in a multidimensional array, you will get back the total number of elements in the array (2 × 100 for our example). To find out the number of elements in a specific dimension, use the GetLength method, passing as an argument a specific dimension. The following expressions will return the number of elements in the two dimensions of the array:
Debug.WriteLine(Employees.GetLength(0))
2
Debug.WriteLine(Employees.GetLength(1))
100
Code language: VB.NET (vbnet)
Because the index of the first array element is zero, the index of the last element is the length of the array minus 1. Let’s say you have declared an array with the following statement to store
player statistics for 15 players, and there are five values per player:
Dim Statistics(14, 4) As Integer
Code language: VB.NET (vbnet)
The following statements will return the highlighted values shown beneath them:
Debug.WriteLine(Statistics.Rank)
2 ' dimensions in array
Debug.WriteLine(Statistics.Length)
75 ' total elements in array
Debug.WriteLine(Statistics.GetLength(0))
15 ' elements in first dimension
Debug.WriteLine(Statistics.GetLength(1))
5 ' elements in second dimension
Debug.WriteLine(Statistics.GetUpperBound(0))
14 ' last index in the first dimension
Debug.WriteLine(Statistics.GetUpperBound(1))
4 ' last index in the second dimension
Code language: VB.NET (vbnet)
Multidimensional arrays are becoming obsolete because arrays (and other collections) of custom structures and objects are more flexible and convenient.