One set of interesting properties of the TextBox control are the autocomplete properties. Have you noticed how Internet Explorer prompts you with possible matches as soon as you start typing an address or your username in a text box (or in the address bar of the browser)? You can easily implement such boxes with a single-line TextBox control and the autocomplete properties.
Basically, you have to tell the TextBox control how it should prompt the user with strings that match the characters already entered on the control and where the matches will come from. Then, the control can display in a drop-down list the strings that begin with the characters already typed by the user. The user can either continue typing (in which case the list of options becomes shorter) or select an item from the list. The autocomplete properties apply to single-line TextBox controls only; they do not take effect on multiline TextBox controls.
In many cases, an autocomplete TextBox control is more functional than a ComboBox control, and you should prefer it. You will see that the ComboBox also supports the autocomplete properties, because they make the control so much easier to use only with the keyboard.
The AutoCompleteMode property determines whether, and how, the TextBox control will prompt users, and its setting is a member of the AutoCompleteMode enumeration (AutoSuggest, AutoAppend, AutoSuggestAppend, and None). In AutoAppend mode, the TextBox control selects the first matching item in the list of suggestions and completes the text. In AutoSuggestAppend mode, the control suggests the first matching item in the list, as before, but it also expands the list.
In AutoSuggest mode, the control simply opens a list with the matching items but doesn’t select any of them. Regular TextBox controls have their AutoCompleteMode property set to False.
The AutoCompleteSource property determines where the list of suggestions comes from; its value is a member of the AutoCompleteSource enumeration, which is shown in Table 4.2.
Member | Description |
---|---|
AllSystemSources | The suggested items are the names of system resources. |
AllUrl | The suggested items are the URLs visited by the target computer. Does not work if you’re deleting the recently viewed pages. |
CustomSource | The suggested items come from a custom collection. |
FileSystem | The suggested items are filenames. |
HistoryList | The suggested items come from the computer’s history list. |
RecentlyUsedList | The suggested items come from the Recently Used folder. |
None | The control doesn’t suggest any items. |
To demonstrate the basics of the autocomplete properties, I’ve included the AutoComplete-TextBoxes project, whose main form is shown in Figure 4.3. This project allows you to set the autocomplete mode and source for a single-line TextBox control. The top TextBox control uses a custom list of words, while the lower one uses one of the built-in autocomplete sources (file system, URLs, and so on).
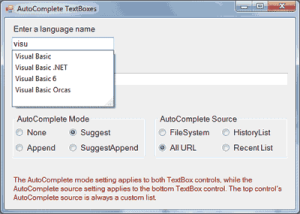
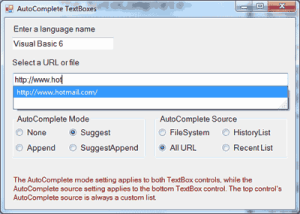
Figure 4.3 – Suggesting words with the AutoComplete-Source property
If you set the AutoCompleteSource to CustomSource, you must also populate an AutoCompleteStringCollection object with the desired suggestions and assign it to the AutoCompleteCustomSource property. The AutoCompleteStringCollection is just a collection of strings. Listing 4.9 shows statements in a form’s Load event that prepare such a list and use it with the TextBox1 control.
Listing 4.9: Populating a Custom AutoCompleteSource Property
Private Sub Form1_Load(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles _
MyBase.Load
' Set up Autocomplete properties for the top TextBox
Dim knownWords As New AutoCompleteStringCollection
knownWords.Add("Visual Basic Orcas")
knownWords.Add("Visual Basic .NET")
knownWords.Add("Visual Basic 6")
knownWords.Add("Visual Basic")
knownWords.Add("Framework")
TextBox1.AutoCompleteCustomSource = knownWords
TextBox1.AutoCompleteSource = AutoCompleteSource.CustomSource
TextBox1.AutoCompleteMode = AutoCompleteMode.Suggest
' Set up Autocomplete properties for the bottom TextBox
TextBox2.AutoCompleteSource = AutoCompleteSource.RecentlyUsedList
TextBox2.AutoCompleteMode = AutoCompleteMode.Suggest
End Sub
Code language: VB.NET (vbnet)
The TextBox1 control on the form will open a drop-down list with all possible matches in the knownWords collection as soon as the user starts typing in the control, as shown in the top part of Figure 4.3. To see the autocomplete properties in action, open the AutoCompleteTextBoxes project and examine its code. The main form of the application, shown in Figure 4.3, allows you to change the AutoCompleteMode property of both TextBox controls on the form, and the AutoComplete-Source property of the bottom TextBox control. The first TextBox uses a list of custom words, which is set up when the form is loaded, with the statements in Listing 4.9.