Variables are storage locations that have a name. Said another way variables are name value pairs. You can assign values to a variable and recall those values by the variable name. To assign a value to a variable use the ‘=’ sign. The format is,
variable name = value
Code language: Python (python)
Here we have an example where the value of Apple is assigned to the variable called fruit.
fruit = 'Apple'
Code language: Python (python)
You can change the value of the variable by reassigning it. Below you can see how to set the value of the fruit variable to the value of orange.
fruit = 'Orange'
Code language: Python (python)
Note that there is nothing significant about the variable name fruit. We could have easily used almost any other variable name that you can think of. When choosing a variable name pick something that represents the data of the variable will hold.
You may know what a variable name X represents today, but if you come back to the code a few months from now you may not. However, if you encounter a variable name fruit chances are you can guess what type of data it will hold.
Variable names are case sensitive. A variable name fruit that begins with a capital F and another variable name fruit that is in all lowercase are two different variables. By convention variables are in all lowercase letter but it’s not a requirement.
Variable names must start with the letter. They can contain numbers but variable names cannot start with a number.
You can also use the underscore character in variable names. You cannot use +, – and some other various symbols in variable names. Whenever you get the urge to use a hyphen just use an underscore (_) instead.
Here are some examples of valid variable names.
first5letters = 'ABCDE'
first_five_letters = 'ABCDE'
firstFiveLetters = 'ABCDE'
Code language: Python (python)
The first example is in all lowercase letters and it contains a number. However, it doesn’t start with a number so it is a valid variable name.
The second line first_five_letters is in all lowercase and it includes underscores which are valid. However, you can’t use dashes so first-five-letters would not be a valid variable name.
The third variable is firstFiveLetters and it’s in mixed case some lowercase letters and some uppercase letters. Again this is perfectly fine, but just note that this variable would be a completely distinct from all lowercase variable name firstfiveletters for example.
In the Python interactive shell, you can run arithmetic operations without declaring it just as shown below.
>>> 25 + 37
62
>>> 15 / 3
5.0
>>> 12 * 9
108
>>> 5 * 4 +3
23
>>> 25 / 5 -2
3.0
>>> 5 * (4 + 3)
35
Code language: Python (python)
In the vast majority of applications that you create and even in interactive mode you’re frequently going to want to store values for later use in your programs. The way that you do that is with variables just like in algebra. For example I can go ahead and create a variable with a simple statement like the one below.
x = 6
Code language: Python (python)
The name of the variable that I have created is x and the value that I have given to x is the integer value 6.
In python anytime you see a whole number like 6 or 25 or 37, that is going to represent an integer value. Whenever you see a number that has a decimal point in it that’s going to represent a floating point value. And python just knows that as part of the languages definition.
Unlike up above when I execute this snippet there is no output for an assignment statement, so this is actually a statement in python that gives the value 6 to the variable x.
>>> x = 6
>>>
Code language: Python (python)
Some place in memory the value 6 is stored and we can now refer to that memory location as x and get the value at that location.
Python is a dynamically typed language so it figures out the type of this variable, not based on how you define it but based on what you assign to it.
So you give it the integer value 6 and python knows that at this moment in time x is an integer with the value 6. But because python is dynamically typed we can actually change that at a later time. We’ll learn that to you as we proceed through this tutorial.
So this is an assignment statement and similarly we could create another variable, we’ll call it y and let’s give it the value 3 here.
>>> y = 3
Code language: Python (python)
Now we have a couple of variables that we can use in the subsequent snippets of code.
Variables in algebra can be used in larger expressions. For example, we can do things like,
>>> x + y
Code language: Python (python)
which is going to look up the value of x, look up the value of y, and then calculate the result. As we have declared above that x is 6, y is 3, so this is really 6+3. And when I press enter you can see that the Python environment immediately figures that out and displays the result for me.
>>> x + y
9
Code language: Python (python)
The great thing about working in python interactive mode in this manner is that you can quickly see your results for every single statement or expression that you write. This enables you to really experiment with the language and learn the language very quickly.
Now, of course when we do an expression like x plus y we might want to store the result for later use. For that let’s go ahead and create a total variable and we’ll give it the value of x plus y.
>>> total = x + y
Code language: Python (python)
so total gets us assigned the value of the expression x plus y, whenever you have an assignment expression or an assignment statement like this, whatever’s on the right side of the assignment is going to be evaluated first, and then the result will be stored into the variable on the left side of the assignment.
The type of the variable total will be determined by the type of evaluating the expression we have x which is the integer 6, we have y which is the integer 3. So when you add two integers you get an integer result and thus total also will be an integer. And at this point if I hit enter we see no output.
>>> total = x + y
>>>
Code language: Python (python)
But in the python interactive session, you can always evaluate a variable to see what its current value is. So I can go ahead and type total and see that indeed total contains the value 9.
>>> total
9
Code language: Python (python)
Variables x, y and total and even the numbers 25, 37, 6 and 3, all of these things have types associated with them. And it turns out that there is a bunch of so called built in functions in the python language that you can use. One of which is a function named ‘type’, which you can give it a value or a variable name and it will tell you the type of that value or variable.
If we want to determine what the type of the variable x is, we can just go ahead and type
>>> type(x)
Code language: Python (python)
And when I press enter here you’ll notice that it displays the type int, which is integer in the python language.
>>> type(x)
<class 'int'>
Code language: Python (python)
If I go ahead and type something like 15.3 inside the parentheses of the type function and press enter we can see that the type of that value is a float.
>>> type (15.3)
<class 'float'>
Code language: Python (python)
So at any point if you have a variable and you’re unsure of what its type is, and you’re working in a python interactive mode, you can simply pass that variables name to the type function and in turn it will give you back the corresponding type name.
I mentioned that type is a built in function. And also in order to know about all of the different built in functions that are available to you as part of the python language, go to https://docs.python.org/3/library/functions.html.
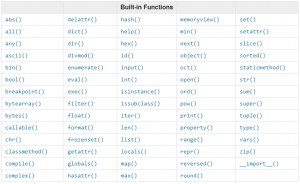
As you can see that there’s quite a number of built in functions available. And in python they tend to take the approach of providing as much as capability as possible to you so that you can focus on the larger task at hand.
You must be logged in to post a comment.