In this lesson, we’re going to take a look at using the IPython interactive mode and for this purpose, we’ll use it as a very simple calculator. There are a few things that we want to present as part of this lesson. The key one is how to execute snippets of code in this interactive mode, in which you’re going to see immediate results as you execute each snippet of code. The mechanism that enables that to work is known as a REPL – read, eval, print, loop.
Basically what’s going to happen is you’ll type some code, then press enter. The REPL, or IPython specifically, will read in the code that you type, evaluate that code, print the result, then display a new prompt at which you can enter more code and repeat that process, thus the concept of the loop.
Many languages are now offering REPLs to enable you to do this kind of interactivity. For example, if you’re coming over from JAVA you might be familiar with the Jshell tool. Microsoft now has REPLs available for certain languages and there are lots of other languages that provide REPLs as well.
in order to work with IPython interactive mode, we’re going to have to open a command line window for your particular operating system. If you’re working with macOS you’ll use the Terminal App which you’ll find in the Applications folder’s Utilities sub folder. On Windows like me, you’re going to want to open the Anaconda Prompt from your Start menu. If you’re on Linux, this is going to depend on your particular distribution of Linux. You’ll either open a Terminal window if you have one or you can simply work with any shell window as well.
We are gong to use the command ipython
to launch the IPython interpreter. The first time you launch it sometimes it takes a few seconds extra. The text that gets displayed will be dependent on the version of Anaconda that you installed. So as of right now, the version of Python that’s installed on my system is Python 3.8.8. And then separately we’re also working with IPython the interpreter version 7.22.0 at this point.
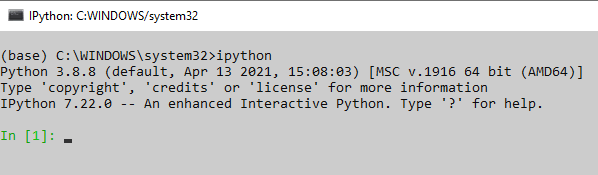
Once you launch the IPython interpreter, the stuff that you see in your command line window is going to basically be the same across all three operating systems. The coloring might be a little bit different, the fonts might be a little bit different, but the results should be the same. With a few minor exceptions, for example, floating point numbers may be represented a little bit differently across systems, for example, numbers like 6.3.
When you start working with floating point arithmetic, there can be slight rounding errors so you may see slight differences in numeric values as you do calculations. Mostly in the digits very far to the right of the decimal point. But other than that, you should see the same results as I show you across all the examples that we present.
What you see in the above figure is known as the In [ ]
prompt. Every time you see an In [ ]
prompt you are capable of typing a snippet of code to execute. For example, let’s say we want to do a simple arithmetic expression we want to add the numbers 54 and 63.
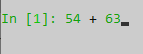
Python, like all programming languages, has arithmetic operators, and for the most part, those look like the same operators that you’re used to in math. But we have to write expressions in programming languages in straight-line form. Meaning left to right across the statement or snippet of code that we’re typing.
If I press enter you can see the immediate feedback is presented in an out prompt. The numbering is the snippet number, so for snippet number one we executed the expression 54 plus 63 and the result of that was 117, displayed in an out prompt for that same snippet number. That’s a very simple arithmetic expression, and of course, we can do more complex ones as well.
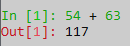
Let’s say we wanted to do the following calculation.
5 * ( 14.7 - 6) / 2
Code language: Python (python)
Before I execute this for those of you who may be less familiar with how math works in the context of a programming language. Operators have precedence, just like they do in regular arithmetic. The multiplication and division operators don’t look like what you might expect if you’re not used to doing programming. Those are of course different symbols because the symbols we use have to be on the keyboard in order to write arithmetic expressions. But just like in regular math, parenthesis may be used to force the order of evaluation. So subtraction has lower precedence than multiplication and division therefore if we want to perform the subtraction first, we have to wrap that in parenthesis.
So 14.7 minus 6 is going to give us 8.7, then we’ll multiply that by five, and divide the result by two. The multiplication and division operators have the same level of precedence and in all but one case in Python operators evaluate left to right. So you do the multiplication in this case before the division. We’ll talk more about operator precedence in the coming lessons when we show you all the arithmetic operators and discuss them in a little bit more detail.
When I go ahead and press enter, I immediately see the result, 21.75.
In [2]: 5 * (14.7 - 6) / 2
Out[2]: 21.75
Code language: Python (python)
Just as a point of emphasis, the forcing of the subtraction to happen first with parenthesis we can see that we get different results if we remove those parenthesis. If you want to re execute a snippet that you did previously you can use your up arrow key to recall that previous snippet. You can also use the down arrow key to go in the opposite direction.
let’s say I wanna re execute the snippet without the parenthesis I can use my left and right arrow keys to work my way through the expression, and then use the delete or backspace key to remove characters.
5 * 14.7 - 6 / 2
Code language: Python (python)
Here’s the same expression without the parenthesis and in this case the multiplication will happen first, the division will happen second, and then we’ll subtract the result. So we’ll do 5 times 14.7, we’ll do 4 divided by 2, and then we’ll subtract from the result of 5 times 14.7 the result of this division which is 3 and see the final result which in this case is 70.5.
In [3]: 5 * 14.7 - 6 / 2
Out[3]: 70.5
Code language: Python (python)
When you’re done with a IPython session, you can exit that session a couple of different ways. You can actually type exit
and press enter and you’ll be returned to your systems command prompt. Separately you can use ctrl + D
at your command prompt to terminate the IPython session. If I type ctrl
+ D once, then you’ll see this prompt do you really want to exit? if you simply press enter the default value yes will be input automatically for you and IPython will terminate and you can also type a Y.
Do you really want to exit ([y]/n)? y
(base) C:\WINDOWS\system32>
Code language: PHP (php)
For those of you who are on macOS or Linux you can type control D twice and it will immediately exit the IPython interpreter.
I just want to point out a couple of minor things about the expressions that we looked at up above here. Anytime you see a whole number value like 5 or 6 in Python, that is what we refer to as an integer value. Anytime you see a number with a decimal point in it, like 14.7, that’s what we refer to as a floating point number. As you’ll see there are types associated with data that you use in your Python applications.
Although, in Python, you typically don’t explicitly specify those types so Python knows that a whole number is an integer or the actual data type is called the int
. And it knows that 14.7 is a floating point number, and the actual data type is called float
. And we’ll be talking about int
and float
and other data types in more detail in the coming lessons.
Executing Python programs using the IPython Interpreter
In this section, I’m going to demonstrate executing a Python program using the Ipython Interpreter. In particular, we’ll be working with the Ipython Interpreter’s script mode. This is the mode in which you tell the Ipython Interpreter the name of the file to execute. The interpreter is going to load that file into memory, it’s going to compile the code from Python source code into Python byte codes, which is an intermediate language in between Python and machine language, which is what actually executes on your computer.
The byte codes execute on a piece of software known as the Python Virtual Machine, which is a layer of software above your operating system that enables Python code to run portably across many different operating systems.
I have already created a hello.py script with the code print ('Hello World!')
in the directory C:\python_work in my Windows computer. We need to be in that folder in order to execute the script. So I am going to go ahead and change it to the desired directory in my Anaconda prompt.
(base) C:\WINDOWS\system32>cd C:\python_work
(base) C:\python_work>
Code language: Python (python)
As you can see, I am in the C:\python_work directory. On Mac Os and Linux, you can type “ls” to see the contents of that folder. If you’re on Windows, you can type “dir” for directory, and press “Enter” to see the contents of that folder. The Linux and Mac OS command lines are case sensitive, so when you specify file names that contain capital letters, you need to specify those correctly.
Now we can move on to actually executing the script. Just like we did when we were entering Ipython interactive mode, we’re going to use the Ipython command, but in this case, rather than going into interactive mode, we’re going to type the full name of the file that we want to execute and press Enter. This will show the output as shown below.
(base) C:\python_work>ipython hello.py
Hello World!
Code language: Python (python)
In the next lesson we’re going to learn how to write and execute code in a Jupiter Notebook.
You must be logged in to post a comment.