In this section, we’re going to take a look at Python’s arithmetic operators. Below figure just shows the operator symbols, sample algebraic expressions containing those operators, and sample Python expressions as well.
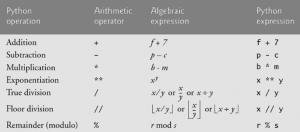
Addition, Subtraction and Multiplication operators
We’ve already demonstrated addition, and subtraction in the earlier section. So I’m gonna focus on the operators that we have not used as yet.
So for example, if I’d like to do some multiplication I can take a couple of values like illustrated below.
>>> 6 * 7
42
Code language: Python (python)
In this case the integers 6 and 7 and separate them with the asterisk (*) operator. And of course you get the result of 42 when you press enter to evaluate that expression.
Exponentiation operator
A nice feature of Python that we don’t have in a lot of the C based languages is the exponentiation operator.
>>> 2 ** 12
4096
Code language: Python (python)
The expression that you’re seeing above is going to raise the value 2 to the 12th power and that’s going to give you the result 4096.
You can use the exponentiation operator with whole number exponents. But you can also use it with fraction exponents as well. For example, if you wanted to take the square root of 25 type
>>> 25 ** (1/2)
5.0
Code language: Python (python)
That will give you the square root of 25 which is of course 5. And I didn’t have to do one divided by two, I also could have done 0.5 and that would work as well.
>>> 25 ** 0.5
5.0
Code language: Python (python)
True Division and Floor Division operators
As we saw in the figure ‘Python’s Arithmetic Operators’ above, we can see that there is a couple of different types of divisions. You have true division which is going to divide a numerator by a denominator and always give you a floating point result. And then you also have floor division, which when you divide a numerator by a denominator give you back the highest integer value that is not greater than the result.
Let’s talk about and demonstrate these two concepts. So we’ll start with the true division operator. Let’s say for example that we want to divide 9 by 5. In Python the true division operator is a forward slash character. And when 9 divided by 5, 5 does not go into 9 a whole number of times so we’re going to get a floating point result.
>>> 9 / 5
1.8
Code language: Python (python)
Here we have 1.8 as the result of the true division. Now if we do that same expression with floor division what happens is it effectively truncates the floating point part of a positive number. And gives you the highest integer value that is not greater than the result.
The result with regular division is 1.8, so the highest integer value that’s not greater than dividing 9 by 5
which gives you 1.8 normally, is going to be the value 1. And you can see we get the whole number 1 as a result out of that.
>>> 9 // 5
1
Code language: Python (python)
If I divide a number by a denominator that’s bigger than the numerator, in this case you’re going to get zero as the result.
>>> 4 // 6
0
Code language: Python (python)
It’s because there’s only a floating point result of a smaller number divided by a bigger number and the biggest integer is not greater than that. So result is going to be value 0.
If I want to do something like 25 divided by 5, of course in this case you get a whole number of times that 5 goes into 25. As a result you get what you would expect, which is the value 5.
>>> 25 // 5
5
Code language: Python (python)
When you’re dealing with negative numbers you may not get exactly what you expect based on what you just saw here. Again you get in floor division the highest value not greater than the result. So let’s take a look at what we get when we work with negative numbers.
If I do true division for -17 divided by 4, we can see in true division the result is -4.25.
>>> -17 / 4
-4.25
Code language: Python (python)
If I do the same expression using the floor division operator, you can see that the result is -5.
>>> -17 // 4
-5
Code language: Python (python)
This maybe something that you don’t really understand based on what’s going on here. But again largest value not greater than the result. So if we were to say -4, it is actually a larger value than -4.25. It actually gives you the largest value not greater than that which is actually -5. It’s going down to the highest integer that is not greater than the value you would get with the true division operator.
Python does not allow division by zero. In Java and C#, and some other languages. when you divide by zero in integer arithmetic, typically you get what is know as an exception. But when you do floating point arithmetic and you divide by zero you get positive or negative infinity in a lot of languages.
In Python whenever you divide by zero whether it’s floating point arithmetic, or integer arithmetic, you always get a division by zero error. It’s actually called a zero division error. For example,
>>> 155 / 0
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
ZeroDivisionError: division by zero
>>>
Code language: Python (python)
What you’re seeing above is the result of what’s known as an exception that occurred. When an exception occurs in python interactive mode it displays a traceback, which is what you’re seeing here. It tells you the name of the error that occurred which ZeroDivisionError in this case and it says that it’s a traceback.
It also shows you the snippet in which that error occurred. So that was the 155 divide by 0 and it was line number one in that snippet. And then it also rehashes the error name and gives you an error message, in this case indicating that we performed ‘division by zero’.
Remainder (modulo) operator
The remainder operator is going to perform division and give you the remainder after that division. So normally if I divide 23 by 5, 5 goes into 23 four times and there’s a remainder of 3 left over, so what I’m going to get out of this is that remainder value of 3.
>>> 23 % 5
3--
Code language: Python (python)
Now a couple of notes about arithmetic before we move on to the next section. You can always use parentheses to group expression to force order of evaluation.
Let’s say that we want to multiply the value 15 by the quantity 4 + 5.
>>> 15 * (4 + 5)
135
Code language: Python (python)
By using the grouping parentheses here to group together the operands 4 and 5 with the plus operator, going to force that expression to execute first. So 4 + 5 gives me 9. And then we’ll multiply that by 15, and if I hit enter you can see the result of 135 in that case.
And of course if we do the same exact expression without the parentheses here, the operator precedence is going to take hold. Multiplication has higher precedence than addition.
>>> 15 * 4 + 5
65
Code language: Python (python)
So in this case we get 15 times 4 which is 60, and then we add the value 5 to that to get the result of 65.
As you can see, you have precedence of the operators which is very similar to what you have in regular arithmetic in algebra for example. And you have parentheses that you can always use to help you force order of evaluation or just to help you clarify an expression if you would like.
You must be logged in to post a comment.