The StringBuilder class doesn’t provide as many methods as the String class. It’s used primarily to build long strings and manipulate them dynamically. If you want to locate words or other patterns in the text, align strings in fixed-length fields, and perform other similar operations, use the String class. We frequently combine both classes in an application: the StringBuilder class for its speed and the String class for its manipulation methods. To extract the text from a StringBuilder, use its ToString method. To assign a string to the StringBuilder variable, use its Append method:
Dim strB As New StringBuilder
Dim str1, str2 As String
str1 = "some text"
strB.Append(str1)
' statements to process the strB variable
str2 = strB.ToString
Code language: PHP (php)
The ToString method of the StringBuilder class returns a string, which can be processed with the methods of the String class. For instance, the StringBuilder class lacks the IndexOf and LastIndexOf methods. To locate an instance of a word in a StringBuilder variable, use the following statement, where SB is a properly declared and initialized StringBuilder variable and pos is the index of the first instance of the word visual in the StringBuilder’s text:
pos = SB.ToString.IndexOf("visual")
Code language: JavaScript (javascript)
The CountWords application, shown in Figure 9.2, counts all instances of a user-supplied word in a StringBuilder variable. You can do the same with the String class, but if you want to further process the text, you’ll have to use the StringBuilder class anyway.
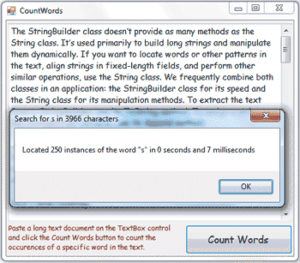
The program prompts the user for a string and attempts to locate it in the text by using the following statement:
startIndex = SB.ToString.ToUpper.IndexOf(searchWord.ToUpper)
The preceding statement performs the search operation by using the uppercase of the string and the search argument to avoid mismatches due to the casing of the strings. The sample project sets up a loop that locates one instance of the user-supplied word at a time. The following statement searches for the word in the text, starting at the location startIndex + searchWord.Length + 1.
This expression is the location of the first character following the most recently located instance of the search argument in the text. At each iteration of the loop, the IndexOf method starts searching for the word in the text following the previous instance of the word. Here’s the statement that locates the next instance of the word in the text:
startIndex = _
SB.ToString.ToUpper.IndexOf(searchWord.ToUpper, _
startIndex + searchWord.length + 1)
This statement appears in a loop that’s repeated for as long as the startIndex variable is positive.When all instances of the word in the text have been located, the IndexOf method returns the value −1 and the loop terminates. The complete code of the Count Words button is shown in Listing 9.6.
Listing 9.6: The CountWords Project’s Code
Dim SB As New System.[Text].StringBuilder()
Dim searchWord As String
searchWord = InputBox( _
"Please enter the word to search for", _
"StringBuilder Search Example", "BASIC")
Dim startIndex As Integer
SB.Append(Textbox1.Text)
startIndex = _
SB.ToString.ToUpper.IndexOf(searchWord.ToUpper)
Dim count As Integer
If startIndex = -1 Then
MsgBox("No instances of the string found")
End If
While startIndex >= 0 And startIndex + _
searchWord.Length < SB.Length
count = count + 1
startIndex = SB.ToString.ToUpper.IndexOf( _
searchWord.ToUpper, _
startIndex + searchWord.Length + 1)
End While
Dim msg As String
msg = "Located " & count.ToString & _
" instances of the word " & searchWord & _
""" in the text"
MsgBox(msg)
End Sub
Code language: PHP (php)
When executed, this code will pop up a message box with a statement like the following:
Located 22 instances of the word in 270 milliseconds
The last few statements calculate the time it took the program to locate all the instances of the word with the methods of the TimeSpan object, which is discussed in the following section.