The Num2String method is far more complicated, but if you can implement it as a regular function, it doesn’t take any more effort to turn it into a method. The listing of Num2String is shown in Listing 6.23. First, it formats the billions in the value (if the value is that large); then it formats the millions, thousands, units, and finally the decimal part, which can contain no more than two digits.
Listing 6.23: Converting Numbers to Strings
Public Function Num2String(ByVal number As Decimal) As String
Dim biln As Decimal, miln As Decimal, thou As Decimal, hund As Decimal
Dim strNumber As String = ""
If number > 999999999999.99 Then
Num2String = "***"
Exit Function
End If
biln = Math.Floor(number / 1000000000)
If biln > 0 Then strNumber = FormatNum(biln) & " Billion" & Pad()
miln = Math.Floor((number - biln * 1000000000) / 1000000)
If miln > 0 Then strNumber = strNumber & FormatNum(miln) & " Million" & Pad()
thou = Math.Floor((number - biln * 1000000000 - miln * 1000000) / 1000)
If thou > 0 Then strNumber = strNumber & FormatNum(thou) & " Thousand" & Pad()
hund = Math.Floor(number - biln * 1000000000 - miln * 1000000 - thou * 1000)
If hund > 0 Then strNumber = strNumber & FormatNum(hund)
If Right(strNumber, 1) = "," Then strNumber = Left(strNumber, Len(strNumber) - 1)
If Left(strNumber, 1) = "," Then strNumber = Right(strNumber, Len(strNumber) - 1)
If number <> Math.Floor(number) Then
strNumber = strNumber & FormatDecimal((number - Math.Floor(number)) * 100)
Else
' the following loop is necessary for the proper conversion
' of amount like 32,100,000 or 1,000,000,000.
While strNumber.EndsWith(", ")
strNumber = Left(strNumber, strNumber.Length - 2) & " "
End While
strNumber = strNumber & " DOLLARS"
End If
Num2String = Delimit(SetCase(strNumber))
End Function
Code language: PHP (php)
Each group of three digits (million, thousand, and so on) is formatted by the FormatNum() function. Then the appropriate string is appended (Million, Thousand, and so on). The FormatNum() function, which converts a numeric value less than 1,000 to the equivalent string, is shown in Listing 6.24.
Listing 6.24: The FormatNum() Function
Private Function FormatNum(ByVal num As Decimal) As String
Dim digit100 As Decimal, digit10 As Decimal
Dim strNum As String = ""
digit100 = Math.Floor(num / 100)
If digit100 > 0 Then strNum = Format100(digit100)
digit10 = Fix((num - digit100 * 100))
If digit10 > 0 Then
If strNum <> "" Then
strNum = strNum & " And " & Format10(digit10)
Else
strNum = Format10(digit10)
End If
End If
Return strNum
End Function
Code language: PHP (php)
The FormatNum() function formats a three-digit number as a string. To do so, it calls the Format100() function to format the hundreds, and the Format10() function formats the tens. The Format10() function calls the Format1() function to format the units. I will not show the code for these functions; you can find it in the StringTools project. You’d probably use similar functions to implement the Num2String method as a function. Instead, I will focus on a few peripheral issues, such as the enumerations used by the class as property values.
To make the Num2String method more flexible, the class exposes the Case, Delimiter, and Padding properties. The Case property determines the case of the characters in the string returned by the method. The Delimiter property specifies the special characters that should appear before and after the string. Finally, the Padding property specifies the character that will appear between groups of digits. The values each of these properties can take on are members of the appropriate enumeration:
PaddingEnum | DelimiterEnum | CaseEnum |
---|---|---|
paddingCommas | delimiterNone | caseCaps |
paddingSpaces | delimiterAsterisk | caseLower |
paddingDashes | delimiter3Asterisks | caseUpper |
The values under each property name are implemented as enumerations, and you need not memorize their names. As you enter the name of the property followed by the equal sign, the appropriate list of values will pop up, and you can select the desired member. Listing 6.25 presents the UseCaseEnum enumeration and the implementation of the UseCase property.
Listing 6.25: The CaseEnum Enumeration and the UseCase Property
Enum CaseEnum
CaseCaps
CaseLower
CaseUpper
End Enum
Private varUseCase As CaseEnum
Public Property [Case]() As CaseEnum
Get
Return (varUseCase)
End Get
Set
varUseCase = Value
End Set
End Property
Code language: PHP (php)
Notice that the name of the Case property is enclosed in square brackets. This is necessary when you’re using a reserved keyword as a variable, property, method, or enumeration member name. Alternatively, you can use a different name for the property to avoid the conflict altogether. After the declaration of the enumeration and the Property procedure are in place, the coding of the rest of the class is simplified a great deal. The Num2String() function, for example, calls the Pad() method after each three-digit group. The separator is specified by the UseDelimiter property, whose type is clsPadding. The Pad() function uses the members of the UsePaddingEnum enumeration to make the code easier to read. As soon as you enter the Case keyword, the list of values that can be used in the Select Case statement will appear automatically, and you can select the desired member. Here’s the code of the Pad() function:
Private Function Pad() As String
Select Case varUsePadding
Case PaddingEnum.PaddingSpaces : Return " "
Case PaddingEnum.PaddingDashes : Return "-"
Case PaddingEnum.PaddingCommas : Return ", "
Case Else : Return Nothing
End Select
End Function
Code language: JavaScript (javascript)
To test the StringTools class, create a test form like the one shown in Figure 6.8. Then enter the code from Listing 6.26 in the Click event handler of the two buttons.
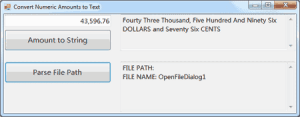
Figure 6.8 – The test form of the StringTools class
Listing 6.26: Testing the StringTools Class
Private Sub bttnAmount2String_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles bttnAmount2String.Click
TextBox1.Text = Convert.ToDecimal(TextBox1.Text).ToString("#,###.00")
Dim objStrTools As New StringTools()
objStrTools.Case = StringTools.CaseEnum.CaseCaps
objStrTools.Delimiter = StringTools.DelimitEnum.DelimiterNone
objStrTools.Padding = StringTools.PaddingEnum.PaddingCommas
TextBox2.Text = objStrTools.Num2String(CDec(TextBox1.Text))
End Sub
Private Sub bttnParsePath_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles bttnParsePath.Click
Dim objStrTools As New StringTools()
OpenFileDialog1.ShowDialog()
TextBox3.Clear()
TextBox3.AppendText("FILE PATH: " & objStrTools.ExtractPathName(OpenFileDialog1.FileName) & vbCrLf)
TextBox3.AppendText("FILE NAME: " & objStrTools.ExtractFileName(OpenFileDialog1.FileName))
End Sub
Code language: PHP (php)