Let’s add some functionality to our bare-bones class. We’ll begin by adding two trivial properties and two methods to perform simple operations. The two properties are called strProperty (a string) and dblProperty (a double). To expose these two members as properties, you can simply declare them as Public variables. This isn’t the best method of implementing properties, but it really doesn’t take more than declaring something as Public to make it available to code outside the class. The following statement exposes the two properties of the class:
Public strProperty As String, dblProperty As Double
Code language: VB.NET (vbnet)
The two methods we’ll implement in our sample class are the ReverseString and Negate Number methods. The first method reverses the order of the characters in strProperty and returns the new string. The NegateNumber method returns the negative of dblProperty. They’re two simple methods that don’t accept any arguments; they simply operate on the values of the properties. Methods are exposed as Public procedures (functions or subroutines), just as properties are exposed as Public variables. Enter the function declarations of Listing 6.1 between the Class Minimal and End Class statements in the class’s code window. (I’m showing the entire listing of the class here.)
Listing 6.1: Adding a Few Members to the Minimal Class
Public Class Minimal
Public strProperty As String, dblProperty As Double
Public Function ReverseString() As String
Return (StrReverse(strProperty))
End Function
Public Function NegateNumber() As Double
Return (-dblProperty)
End Function
End Class
Code language: VB.NET (vbnet)
Let’s test the members we’ve implemented so far. Switch back to your form and enter the lines shown in Listing 6.2 in a new button’s Click event handler. The obj variable is of the Minimal type and exposes the Public members of the class. You can set and read its properties, and call its methods. In Figure 6.2, you see a few more members than the ones added so far; we’ll extend our Minimal class in the following section. Your code doesn’t see the class’s code, just as it doesn’t see any of the built-in classes’ code. You trust that the class knows what it is doing and does it right.
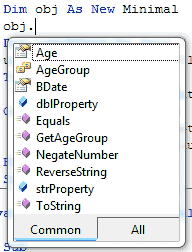
Figure 6.2 – The members of the class are displayed automatically by the IDE, as needed.
Listing 6.2: Testing the Minimal Class
Dim obj As New Minimal
obj.strProperty = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"
obj.dblProperty = 999999
Debug.WriteLine(obj.ReverseString)
Debug.WriteLine(obj.NegateNumber)
Code language: VB.NET (vbnet)
Every time you create a new variable of the Minimal type, you’re creating a new instance of the Minimal class. The class’s code is loaded into memory only the first time you create a variable of this type, but every time you declare another variable of the same type, a new set of variables is created. This is called an instance of the class. The code is loaded once, but it can act on different sets of variables. In effect, different instances of the class are nothing more than different sets of local variables.
The New Keyword
The New keyword tells VB to create a new instance of the Minimal class. If you omit the New keyword, you’re telling the compiler that you plan to store an instance of the Minimal class in the obj variable, but the class won’t be instantiated. All the compiler can do is prevent you from storing an object of any other type in the obj variable. You must still initialize the obj variable with the New keyword on
a separate line:
obj = New Minimal
It’s the New keyword that creates the object in memory. The obj variable simply points to this object. If you omit the New keyword, a Null Reference exception will be thrown when the code attempts to use the variable. This means that the variable is Nothing — it hasn’t been initialized yet. Even as you work in the editor’s window, the name of the variable will be underlined and the following warning will be generated: Variable ‘obj’ is used before it has been assigned a value. A null reference exception could result at runtime. You can compile the code and run it if you want, but everything will proceed as predicted: As soon as the statement that produced the warning is reached, the runtime exception will be thrown.